Canyon Animation
<< Perspective | FinalProjectsTrailIndex | Sound Stuff >>
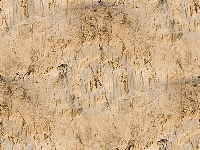
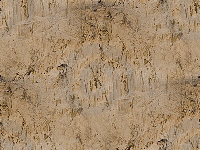
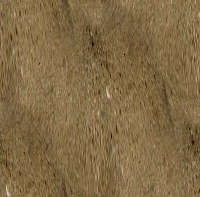
MovingSurface.java
import java.awt.Graphics; import java.awt.Graphics2D; import java.awt.Polygon; import java.awt.TexturePaint; import java.awt.geom.Rectangle2D; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import javax.imageio.ImageIO; public class MovingSurface { public static final int UP=0; public static final int DOWN=1; public static final int LEFT=2; public static final int RIGHT=3; private Polygon p; private int speed,direction; private BufferedImage img; //the texture // see http://oreilly.com/catalog/java2d/chapter/ch04.html public MovingSurface(String graphicFileName,int speed, int direction){ this.p=new Polygon(); this.direction=direction; this.speed=speed; this.img = null; try { img = ImageIO.read(new File(graphicFileName)); } catch (IOException e) {} } public void addPoint(int x, int y){ p.addPoint(x, y); } public int getWidth(){ return img.getWidth();} public int getHeight(){ return img.getHeight();} /** * * @param g the Graphics object to draw on * @param f the frame number used to animate img on the polygon */ public void draw(Graphics g, int f){ Graphics2D g2=(Graphics2D)g; int height=img.getHeight(); int width=img.getWidth(); int i= speed*f % width;// presume right Rectangle2D tr=new Rectangle2D.Double(i,0,width, height);//horiz //now check: if (direction==LEFT){ i= width-(speed*f % width);//left } else if (direction==DOWN){ i= speed*f % img.getHeight();//down tr=new Rectangle2D.Double(0,i,width, height);//vert } else if (direction==UP){ i= height-(speed*f % height);//up tr=new Rectangle2D.Double(0,i,width, height);//vert } TexturePaint tp=new TexturePaint(img,tr); g2.setPaint(tp); g2.fill(p); } }
CanyonAnimation.java
import java.awt.event.*; import java.awt.geom.Rectangle2D; import java.awt.image.BufferedImage; import java.applet.*; import java.awt.*; import java.io.File; import java.io.IOException; import javax.imageio.ImageIO; import javax.swing.*; public class CanyonAnimation extends Applet implements ActionListener { Timer timer; int frame, lastFrame; Image virtualMem; BufferedImage img; Graphics gBuffer; Button startButton; MovingSurface leftWall,rtWall,bottom,bottomRt; //AudioClip racingSound; public void init(){ int width=getWidth(); int height=getHeight(); timer = new Timer(1, this); frame=0; lastFrame=100; virtualMem = createImage(width,height); gBuffer = virtualMem.getGraphics(); gBuffer.setColor(Color.white); gBuffer.fillRect(0,0,width,height); gBuffer.setColor(Color.black); gBuffer.drawLine(width-10,height-frame,width+10,height-frame); startButton= new Button("Start"); //the class is its own button listener startButton.addActionListener (this); add(startButton); //Audio //racingSound = getAudioClip(getDocumentBase(), "racing.wav"); //Initialize side polygons leftWall=new MovingSurface("sand1.gif", -15, MovingSurface.LEFT); leftWall.addPoint(0,0); leftWall.addPoint(300, 100); leftWall.addPoint(300, 500); leftWall.addPoint(0, 600); rtWall=new MovingSurface("sand2.gif", 15, MovingSurface.RIGHT); rtWall.addPoint(800,0); rtWall.addPoint(500, 100); rtWall.addPoint(500, 500); rtWall.addPoint(800, 600); //lastFrame=rtWall.getWidth(); bottom=new MovingSurface("sand3.gif", 10, MovingSurface.DOWN); bottom.addPoint(0,600); bottom.addPoint(300,400); bottom.addPoint(500, 400); bottom.addPoint(800, 600); } public void paint(Graphics g){ int width=getWidth(); int height=getHeight(); //blue sky gBuffer.setColor(Color.white); gBuffer.fillRect(0,0,width,height); //draw sides //cover sides with sky and road leftWall.draw(gBuffer, frame); rtWall.draw(gBuffer, frame); bottom.draw(gBuffer, frame); gBuffer.setColor(Color.black); int deltaX=3*frame; int y=height/2+frame*frame/5; gBuffer.fillRect(width/2-deltaX,y,deltaX,deltaX); //Now we send the result to the screen g.drawImage(virtualMem,0,0,this); } @Override public void actionPerformed(ActionEvent e) { Object source = e.getSource(); if (source == timer) { if (frame < lastFrame) { frame++; } else { frame=0; } } else { //restart button pressed frame=0; if (timer.isRunning()) { timer.stop(); startButton.setLabel("Start"); } else { timer.start(); startButton.setLabel("Stop"); } } repaint(); } public void update(Graphics g) { paint(g); //get rid of flicker with this method } }