Dueling Robots Example
<< Mouse Motion Listener Application | Applications | Button Application >>
Here is some starter code for dueling robots.
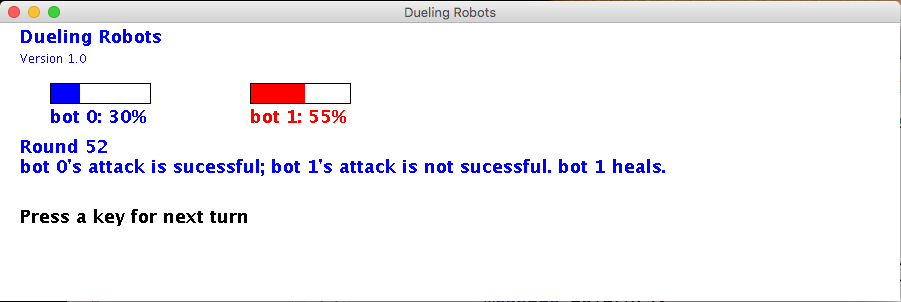
Robot.java
public class Robot { //Probability to defend, hit, and heal private double defense, offense, healing; private int health; private String name; //other ideas for instance fields: // color, teamAffiliation public Robot(String n, double d, double o, double h){ name=n; defense=d; offense=o; healing=h; health=100; } public Robot(){ this("Bot",.1, .75, .1); } public Robot(String n){ this(n,.5, .5, .5); } public String toString(){ return name+": "+health+"%"; } public int health(){ return health; } public String name(){ return name; } public boolean heal(int amt){ boolean result=false; if (Math.random()<healing){ health+=amt; result=true; } return result; } public boolean attack(Robot other){ boolean result=false; if ( Math.random()<offense){ result=other.hit(5); } return result; } private boolean hit(int amt) { boolean result=false; if (Math.random()>defense){ health-=amt; result=true; } return result; } }
DuelingRobots.java
import java.awt.Color; import java.awt.Font; import java.awt.Graphics; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; import javax.swing.JFrame; import javax.swing.JPanel; public class DuelingRobots extends JPanel implements KeyListener { public static int WIDTH=900; public static int HEIGHT=300; private Font titleFont, regularFont; private Robot[] contestant; private String message; private int roundNo; public DuelingRobots() { //initialize variables here... titleFont = new Font("Roman", Font.BOLD, 18); regularFont = new Font("Sans", Font.PLAIN, 12); contestant = new Robot[2]; contestant[0]=new Robot("bot 0"); contestant[1]=new Robot("bot 1"); message="New Game"; roundNo=0; } public static void main(String[] args) { DuelingRobots app= new DuelingRobots(); JFrame window = new JFrame("Dueling Robots"); window.setSize(WIDTH, HEIGHT); window.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); window.getContentPane().add(app); window.addKeyListener(app); window.setVisible(true); } public void paintComponent(Graphics g){ super.paintComponent(g); g.setColor(Color.WHITE); g.fillRect(0, 0, getWidth(),getHeight()); g.setColor(Color.BLUE); g.setFont(titleFont); g.drawString("Dueling Robots", 20, 20); g.drawString(contestant[0].toString(), 50, 100); g.fillRect(50, 60, contestant[0].health(), 20); g.setColor(Color.RED); g.drawString(contestant[1].toString(), 250, 100); g.fillRect(250, 60, contestant[1].health(), 20); g.setColor(Color.BLACK); g.drawString("Press a key for next turn", 20, 200); g.drawRect(50, 60, 100, 20); g.drawRect(250, 60, 100, 20); g.setColor(Color.BLUE); g.drawString("Round "+roundNo, 20, 130); g.drawString(message, 20, 150); g.setFont(regularFont); g.drawString("Version 1.0", 20, 40); g.setColor(Color.MAGENTA); } // update is a workaround to cure Windows screen flicker problem public void update(Graphics g){ paint(g); } // These 3 methods need to be declares to implement the KeyListener Interface @Override public void keyTyped(KeyEvent e) {} @Override public void keyPressed(KeyEvent e) { if(contestant[0].health()>=0 && contestant[1].health()>=0){ message=doTurn(); roundNo++; }else message="Game Over"; repaint(); } private String doTurn() { String result=contestant[0].name()+"'s attack is "; if (!contestant[0].attack(contestant[1]) ){ result+="not "; } result+="sucessful; "+contestant[1].name()+"'s attack is "; if (!contestant[1].attack(contestant[0]) ){ result+="not "; } result+="sucessful. "; for (Robot bot:contestant) if (bot.heal(1)) result+=bot.name()+" heals. "; return result; } @Override public void keyReleased(KeyEvent e) {} }