Elevens Activity 1
<< Elevens Activity 11 | ElevensLab | Elevens Activity 2 >>
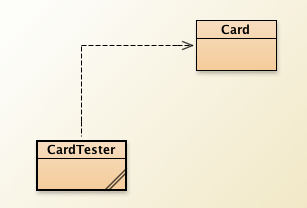
Exercises:
1 Complete the implementation of the provided Card class. You will be required to complete:
A. a constructor that takes two String parameters that represent the card’s rank and suit, and an int parameter that represents the point value of the card;
B accessor methods for the card’s rank, suit, and point value;
C a method to test equality between two card objects; and
D the toString method to create a String that contains the rank, suit, and point value of the card object. The string should be in the following format: rank of suit (point value = pointValue)
2 Once you have completed the Card class, find the CardTester.java file in the Activity1 Starter Code folder. Create three Card objects and test each method for each Card object.
Card.java
/** * Card.java * * <code>Card</code> represents a playing card. */ public class Card { /** * String value that holds the suit of the card */ private String suit; /** * String value that holds the rank of the card */ private String rank; /** * int value that holds the point value. */ private int pointValue; /** * Creates a new <code>Card</code> instance. * * @param cardRank a <code>String</code> value * containing the rank of the card * @param cardSuit a <code>String</code> value * containing the suit of the card * @param cardPointValue an <code>int</code> value * containing the point value of the card */ public Card(String cardRank, String cardSuit, int cardPointValue) { /* *** TO BE IMPLEMENTED IN ACTIVITY 1 *** */ } /** * Accesses this <code>Card's</code> suit. * @return this <code>Card's</code> suit. */ public String suit() { /* *** TO BE IMPLEMENTED IN ACTIVITY 1 *** */ } /** * Accesses this <code>Card's</code> rank. * @return this <code>Card's</code> rank. */ public String rank() { /* *** TO BE IMPLEMENTED IN ACTIVITY 1 *** */ } /** * Accesses this <code>Card's</code> point value. * @return this <code>Card's</code> point value. */ public int pointValue() { /* *** TO BE IMPLEMENTED IN ACTIVITY 1 *** */ } /** Compare this card with the argument. * @param otherCard the other card to compare to this * @return true if the rank, suit, and point value of this card * are equal to those of the argument; * false otherwise. */ public boolean matches(Card otherCard) { /* *** TO BE IMPLEMENTED IN ACTIVITY 1 *** */ } /** * Converts the rank, suit, and point value into a string in the format * "[Rank] of [Suit] (point value = [PointValue])". * This provides a useful way of printing the contents * of a <code>Deck</code> in an easily readable format or performing * other similar functions. * * @return a <code>String</code> containing the rank, suit, * and point value of the card. */ @Override public String toString() { /* *** TO BE IMPLEMENTED IN ACTIVITY 1 *** */ } }
CardTester.java
/** * This is a class that tests the Card class. */ public class CardTester { /** * The main method in this class checks the Card operations for consistency. * @param args is not used. */ public static void main(String[] args) { /* *** TO BE IMPLEMENTED IN ACTIVITY 1 *** */ } }