Elevens Activity 8
<< Elevens Activity 7 | ElevensLab | Elevens Activity 9 >>
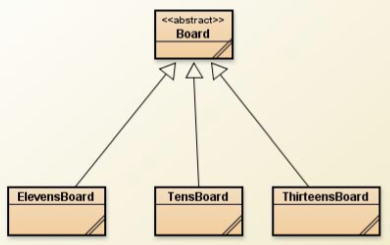
Questions
- Discuss the similarities and differences between Elevens, Thirteens, and Tens.
- As discussed previously, all of the instance variables are declared in the Board class. But it is the ElevensBoard class that “knows” the board size, and the ranks, suits, and point values of the cards in the deck. How do the Board instance variables get initialized with the ElevensBoard values? What is the exact mechanism?
- Now examine the files Board.java, and ElevensBoard.java, found in the Activity8 Starter Code directory. Identify the abstract methods in Board.java. See how these methods are implemented in ElevensBoard. Do they cover all the differences between Elevens, Thirteens, and Tens as discussed in question 1? Why or why not?
Board.java
import java.util.List; import java.util.ArrayList; /** * This class represents a Board that can be used in a collection * of solitaire games similar to Elevens. The variants differ in * card removal and the board size. */ public abstract class Board { /** * The cards on this board. */ private Card[] cards; /** * The deck of cards being used to play the current game. */ private Deck deck; /** * Flag used to control debugging print statements. */ private static final boolean I_AM_DEBUGGING = false; /** * Creates a new <code>Board</code> instance. * @param size the number of cards in the board * @param ranks the names of the card ranks needed to create the deck * @param suits the names of the card suits needed to create the deck * @param pointValues the integer values of the cards needed to create * the deck */ public Board(int size, String[] ranks, String[] suits, int[] pointValues) { cards = new Card[size]; deck = new Deck(ranks, suits, pointValues); if (I_AM_DEBUGGING) { System.out.println(deck); System.out.println("----------"); } dealMyCards(); } /** * Start a new game by shuffling the deck and * dealing some cards to this board. */ public void newGame() { deck.shuffle(); dealMyCards(); } /** * Accesses the size of the board. * Note that this is not the number of cards it contains, * which will be smaller near the end of a winning game. * @return the size of the board */ public int size() { return cards.length; } /** * Determines if the board is empty (has no cards). * @return true if this board is empty; false otherwise. */ public boolean isEmpty() { for (int k = 0; k < cards.length; k++) { if (cards[k] != null) { return false; } } return true; } /** * Deal a card to the kth position in this board. * If the deck is empty, the kth card is set to null. * @param k the index of the card to be dealt. */ public void deal(int k) { cards[k] = deck.deal(); } /** * Accesses the deck's size. * @return the number of undealt cards left in the deck. */ public int deckSize() { return deck.size(); } /** * Accesses a card on the board. * @return the card at position k on the board. * @param k is the board position of the card to return. */ public Card cardAt(int k) { return cards[k]; } /** * Replaces selected cards on the board by dealing new cards. * @param selectedCards is a list of the indices of the * cards to be replaced. */ public void replaceSelectedCards(List<Integer> selectedCards) { for (Integer k : selectedCards) { deal(k.intValue()); } } /** * Gets the indexes of the actual (non-null) cards on the board. * * @return a List that contains the locations (indexes) * of the non-null entries on the board. */ public List<Integer> cardIndexes() { List<Integer> selected = new ArrayList<Integer>(); for (int k = 0; k < cards.length; k++) { if (cards[k] != null) { selected.add(new Integer(k)); } } return selected; } /** * Generates and returns a string representation of this board. * @return the string version of this board. */ public String toString() { String s = ""; for (int k = 0; k < cards.length; k++) { s = s + k + ": " + cards[k] + "\n"; } return s; } /** * Determine whether or not the game has been won, * i.e. neither the board nor the deck has any more cards. * @return true when the current game has been won; * false otherwise. */ public boolean gameIsWon() { if (deck.isEmpty()) { for (Card c : cards) { if (c != null) { return false; } } return true; } return false; } /** * Method to be completed by the concrete class that determines * if the selected cards form a valid group for removal. * @param selectedCards the list of the indices of the selected cards. * @return true if the selected cards form a valid group for removal; * false otherwise. */ public abstract boolean isLegal(List<Integer> selectedCards); /** * Method to be completed by the concrete class that determines * if there are any legal plays left on the board. * @return true if there is a legal play left on the board; * false otherwise. */ public abstract boolean anotherPlayIsPossible(); /** * Deal cards to this board to start the game. */ private void dealMyCards() { for (int k = 0; k < cards.length; k++) { cards[k] = deck.deal(); } } }
ElevensBoard.java
import java.util.List; import java.util.ArrayList; /** * The ElevensBoard class represents the board in a game of Elevens. */ public class ElevensBoard extends Board { /** * The size (number of cards) on the board. */ private static final int BOARD_SIZE = 9; /** * The ranks of the cards for this game to be sent to the deck. */ private static final String[] RANKS = {"ace", "2", "3", "4", "5", "6", "7", "8", "9", "10", "jack", "queen", "king"}; /** * The suits of the cards for this game to be sent to the deck. */ private static final String[] SUITS = {"spades", "hearts", "diamonds", "clubs"}; /** * The values of the cards for this game to be sent to the deck. */ private static final int[] POINT_VALUES = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 0, 0, 0}; /** * Flag used to control debugging print statements. */ private static final boolean I_AM_DEBUGGING = false; /** * Creates a new <code>ElevensBoard</code> instance. */ public ElevensBoard() { super(BOARD_SIZE, RANKS, SUITS, POINT_VALUES); } /** * Determines if the selected cards form a valid group for removal. * In Elevens, the legal groups are (1) a pair of non-face cards * whose values add to 11, and (2) a group of three cards consisting of * a jack, a queen, and a king in some order. * @param selectedCards the list of the indices of the selected cards. * @return true if the selected cards form a valid group for removal; * false otherwise. */ @Override public boolean isLegal(List<Integer> selectedCards) { /* *** TO BE IMPLEMENTED IN ACTIVITY 9 *** */ } /** * Determine if there are any legal plays left on the board. * In Elevens, there is a legal play if the board contains * (1) a pair of non-face cards whose values add to 11, or (2) a group * of three cards consisting of a jack, a queen, and a king in some order. * @return true if there is a legal play left on the board; * false otherwise. */ @Override public boolean anotherPlayIsPossible() { /* *** TO BE IMPLEMENTED IN ACTIVITY 9 *** */ } /** * Check for an 11-pair in the selected cards. * @param selectedCards selects a subset of this board. It is list * of indexes into this board that are searched * to find an 11-pair. * @return true if the board entries in selectedCards * contain an 11-pair; false otherwise. */ private boolean containsPairSum11(List<Integer> selectedCards) { /* *** TO BE IMPLEMENTED IN ACTIVITY 9 *** */ } /** * Check for a JQK in the selected cards. * @param selectedCards selects a subset of this board. It is list * of indexes into this board that are searched * to find a JQK group. * @return true if the board entries in selectedCards * include a jack, a queen, and a king; false otherwise. */ private boolean containsJQK(List<Integer> selectedCards) { /* *** TO BE IMPLEMENTED IN ACTIVITY 9 *** */ } }