Employee
<< Car Talk Lab | LabTrailIndex | Nifty Uno >>
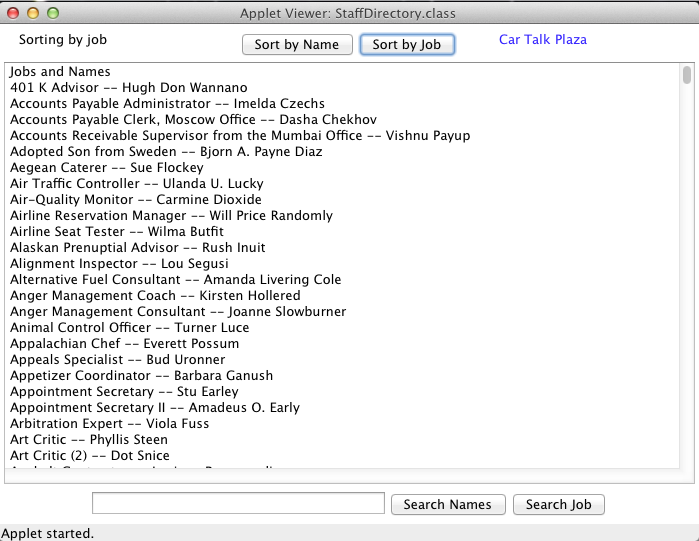
Here is the tab separated file of employees at Car Talk Plaza:
Here is the Empolyee Class:
Employee.java
/** * class Employee holds Infomation about an Employee at Car Talk Plaza. * */ public class Employee { private String job; private String fullName; private String lastName; private String phone; private String SSN; /** * Constructor for objects of class Employee */ public Employee(String j, String n) { job=j; fullName=n; lastName=n.substring(n.lastIndexOf(" ")+1); phone="("+rand(3)+") "+rand(3)+"-"+rand(4); SSN=rand(3)+"-"+rand(2)+"-"+rand(4); } public Employee(String[] s) { job=s[0]; fullName=s[1]; lastName=s[2]; phone=s[3]; SSN=s[4]; } public String getName (){ return fullName; } public String getLastName (){ return lastName; } public String getPhone (){ return phone; } public String getSSN (){ return SSN; } public String getJob (){ return job; } public int rand(int digits){ int result = (int)(10*Math.random()); int d=1; while (d<digits){ result=10*result + (int)(10*Math.random()); d++; } return result; } }
Here is the application class...make sure the datafile is in the same directory as the projects class files:
StaffDirectory.java
import java.io.*; import java.text.DecimalFormat; import java.util.ArrayList; import java.awt.*; import java.awt.event.*; import javax.swing.*; import java.awt.Frame; import java.awt.event.WindowEvent; import java.awt.event.WindowListener; /** * Class StaffDirectory - for browsing and searching Car Talk Employees * employee Information * original Applet version 4 Feb 2009 * * @author Chris Thiel, OFMCap * @version 9 Feb 2023 */ public class StaffDirectory extends Frame implements ActionListener { // instance variables - replace the example below with your own private int x; TextArea textArea; ArrayList<Employee> list; Button sortName; Button sortJob; Button searchName; Button searchJob; TextField searchFor; String message; public StaffDirectory() { super("Car Talk Staff Directory"); setSize(1000,800); this.addWindowListener(new WindowListener() { public void windowClosing(WindowEvent e) {System.exit(0);} public void windowClosed(WindowEvent e) {} public void windowOpened(WindowEvent e) {} public void windowIconified(WindowEvent e) {} public void windowDeiconified(WindowEvent e) {} public void windowActivated(WindowEvent e) {} public void windowDeactivated(WindowEvent e) {} }); list=new ArrayList<Employee>(); textArea=new TextArea("Added the following:",25,70); textArea.setEditable(false); setLayout(new FlowLayout()); sortName = new Button("Sort by Name"); sortName.addActionListener(this); sortJob = new Button("Sort by Job"); sortJob.addActionListener(this); add(sortName); add(sortJob); setSize(650,800); add(textArea); add(new Label("Search for: ")); searchFor = new TextField(30); add(searchFor); searchName = new Button("Search Names"); searchName.addActionListener(this); searchJob = new Button("Search Job"); searchJob.addActionListener(this); add(searchName); add(searchJob); File dataFile = new File("CarTalkStaff.txt"); if (dataFile.exists()) { message="Car Talk Staff"; try{ FileReader inFile = new FileReader(dataFile); BufferedReader inStream = new BufferedReader(inFile); String newLine; newLine = inStream.readLine(); int i=0; while (newLine!=null){ i++; String[] s= newLine.split("\t"); Employee e=new Employee(s); list.add(e); textArea.append("\n"+i+". "+e.getJob()+" -- "+e.getFullName()); newLine = inStream.readLine(); } inStream.close(); textArea.select(0,0); } catch (IOException e){ message="File \"CarTalkStaff.tab\" not Found"; } } } public static void main(String[] args) { StaffDirectory app= new StaffDirectory(); app.setVisible(true); } /** * Paint method for (applet-->application). * * @param g the Graphics object for this applet */ public void paint(Graphics g) { // simple text displayed on applet g.setColor(Color.white); g.fillRect(0, 0, getWidth(), getHeight()); g.setColor(Color.black); g.drawString(message, 20, 20); g.setColor(Color.blue); g.drawString("Car Talk Plaza", 500, 20); } /** * showRecord will return a String describing the * the desired Employee from the list. */ public String showRecord(int i){ String result="Record not found"; if (i>=0 && i<list.size() ){ Employee e=list.get(i); result=e.getJob()+"\n"; result+=e.getName()+"\n"; result+="SSN: "+e.getSSN()+"\n"; result+="Telephone: "+e.getPhone()+"\n"; } return result; } /** * Here is the implementaqtion of the ActionPerformed interface * * This interface requred us to define a specific method called * actionPerformed, which will handle any ActionEvent which * is generated when our user pressed a button. */ public void actionPerformed (ActionEvent ev){ Object source = ev.getSource(); if (source == sortName){ list=mergeSort(list, "lastName"); message="Sorting by last name"; textArea.setText("Names and Jobs"); for (Employee e:list) textArea.append( "\n"+e.getName()+" -- "+e.getJob() ); } if (source == sortJob){ message="Sorting by job"; list=mergeSort(list, "job"); textArea.setText("Jobs and Names"); for (Employee e:list) textArea.append( "\n"+e.getJob()+" -- "+e.getName()); } if (source == searchName){ String userInput = searchFor.getText(); message="Searching Names for "+userInput; textArea.setText(message); list=mergeSort(list, "lastName"); int record =findLastName(userInput,list); textArea.setText(showRecord(record)); } if (source == searchJob){ String userInput = searchFor.getText(); message="Searching Jobs for "+userInput; int record =findJob(userInput,list); textArea.setText(showRecord(record)); } repaint(); } public static ArrayList<Employee> mergeSort(ArrayList<Employee> a, String field) { if (a.size()<=1) return a; int middle=a.size()/2; ArrayList<Employee> left=new ArrayList<Employee>(); ArrayList<Employee> right=new ArrayList<Employee>(); for (int i=0;i<middle; i++) { left.add(a.get(i)); } for (int i=middle;i<a.size();i++) { right.add(a.get(i)); } left =mergeSort(left, field); right=mergeSort(right, field); ArrayList<Employee> result=merge(left,right, field); return result; } private static ArrayList<Employee> merge(ArrayList<Employee> left, ArrayList<Employee> right, String field) { ArrayList<Employee> result=new ArrayList<Employee>(); while (left.size()>0 && right.size()>0) { String theLeft="",theRight=""; if (field.equals("lastName")) { theLeft=(left.get(0)).getLastName(); theRight=(right.get(0)).getLastName(); } if (field.equals("job")) { theLeft=(left.get(0)).getJob(); theRight=(right.get(0)).getJob(); } if (theLeft.compareTo(theRight)<0){ result.add(left.get(0)); left.remove(0); }else { result.add(right.get(0)); right.remove(0); } } //Since left or right ran out, we attach the remainder to the result while (left.size()>0) { result.add(left.get(0)); left.remove(0); } while (right.size()>0) { result.add(right.get(0)); right.remove(0); } return result; } /** * When the search by Name button is pressed it calls this method * it should search the list of Employees for last, * returning the index of the first record it finds with * the search string last, or a -1 if it was not found **/ public int findLastName(String last, ArrayList<Employee> list) { //Your code here return -1; } /** * When the search by Job button is pressed it calls this method * it should search the list of Employees for the job, * returning the index of the first record it finds with * the search string job, or a -1 if it was not found **/ public int findJob(String job, ArrayList<Employee> list) { //Your code here return -1; } }
Here is the old deprecated applet... make sure the data file is in the same directory as the class files:
StaffDirectory.java
import java.io.*; import java.text.DecimalFormat; import java.util.ArrayList; import java.awt.*; import java.awt.event.*; import javax.swing.*; /** * Class StaffDirectory - Applet for browsing and searching * employee Information * * @author Chris Thiel, OFMCap * @version 4 Feb 2009 */ public class StaffDirectory extends JApplet implements ActionListener { // instance variables - replace the example below with your own private int x; TextArea textArea; ArrayList<Employee> list; Button sortName; Button sortJob; Button searchName; Button searchJob; TextField searchFor; String message; public void init() { list=new ArrayList<Employee>(); textArea=new TextArea("Added the following:",25,70); textArea.setEditable(false); setLayout(new FlowLayout()); sortName = new Button("Sort by Name"); sortName.addActionListener(this); sortJob = new Button("Sort by Job"); sortJob.addActionListener(this); add(sortName); add(sortJob); setSize(700,500); add(textArea); searchFor = new TextField(30); add(searchFor); searchName = new Button("Search Names"); searchName.addActionListener(this); searchJob = new Button("Search Job"); searchJob.addActionListener(this); add(searchName); add(searchJob); File dataFile = new File("CarTalkStaff.txt"); if (dataFile.exists()) { message="Car Talk Staff"; try{ FileReader inFile = new FileReader(dataFile); BufferedReader inStream = new BufferedReader(inFile); String newLine; newLine = inStream.readLine(); int i=0; while (newLine!=null){ i++; String[] s= newLine.split("\t"); Employee e=new Employee(s); list.add(e); textArea.append("\n"+i+". "+e.getJob()+" -- "+e.getName()); newLine = inStream.readLine(); } inStream.close(); textArea.select(0,0); } catch (IOException e){ message="File \"CarTalkStaff.tab\" not Found"; } } } /** * Paint method for applet. * * @param g the Graphics object for this applet */ public void paint(Graphics g) { // simple text displayed on applet g.setColor(Color.white); g.fillRect(0, 0, getWidth(), getHeight()); g.setColor(Color.black); g.drawString(message, 20, 20); g.setColor(Color.blue); g.drawString("Car Talk Plaza", 500, 20); } /** * showRecord will return a String describing the * the desired Employee from the list. */ public String showRecord(int i){ String result="Record not found"; if (i>=0 && i<list.size() ){ Employee e=list.get(i); result=e.getJob()+"\n"; result+=e.getName()+"\n"; result+="SSN: "+e.getSSN()+"\n"; result+="Telephone: "+e.getPhone()+"\n"; } return result; } /** * Here is the implementaqtion of the ActionPerformed interface * * This interface requred us to define a specific method called * actionPerformed, which will handle any ActionEvent which * is generated when our user pressed a button. */ public void actionPerformed (ActionEvent ev){ Object source = ev.getSource(); if (source == sortName){ list=mergeSort(list, "lastName"); message="Sorting by last name"; textArea.setText("Names and Jobs"); for (Employee e:list) textArea.append( "\n"+e.getName()+" -- "+e.getJob() ); } if (source == sortJob){ message="Sorting by job"; list=mergeSort(list, "job"); textArea.setText("Jobs and Names"); for (Employee e:list) textArea.append( "\n"+e.getJob()+" -- "+e.getName()); } if (source == searchName){ String userInput = searchFor.getText(); message="Searching Names for "+userInput; textArea.setText(message); list=mergeSort(list, "lastName"); int record =findLastName(userInput,list); textArea.setText(showRecord(record)); } if (source == searchJob){ String userInput = searchFor.getText(); message="Searching Jobs for "+userInput; int record =findJob(userInput,list); textArea.setText(showRecord(record)); } repaint(); } public static ArrayList<Employee> mergeSort(ArrayList<Employee> a, String field) { if (a.size()<=1) return a; int middle=a.size()/2; ArrayList<Employee> left=new ArrayList<Employee>(); ArrayList<Employee> right=new ArrayList<Employee>(); for (int i=0;i<middle; i++) { left.add(a.get(i)); } for (int i=middle;i<a.size();i++) { right.add(a.get(i)); } left =mergeSort(left, field); right=mergeSort(right, field); ArrayList<Employee> result=merge(left,right, field); return result; } private static ArrayList<Employee> merge(ArrayList<Employee> left, ArrayList<Employee> right, String field) { ArrayList<Employee> result=new ArrayList<Employee>(); while (left.size()>0 && right.size()>0) { String theLeft="",theRight=""; if (field.equals("lastName")) { theLeft=(left.get(0)).getLastName(); theRight=(right.get(0)).getLastName(); } if (field.equals("job")) { theLeft=(left.get(0)).getJob(); theRight=(right.get(0)).getJob(); } if (theLeft.compareTo(theRight)<0){ result.add(left.get(0)); left.remove(0); }else { result.add(right.get(0)); right.remove(0); } } //Since left or right ran out, we attach the remainder to the result while (left.size()>0) { result.add(left.get(0)); left.remove(0); } while (right.size()>0) { result.add(right.get(0)); right.remove(0); } return result; } /** * When the search by Name button is pressed it calls this method * it should search the list of Employees for last, * returning the index of the first record it finds with * the search string last, or a -1 if it was not found **/ public int findLastName(String last, ArrayList<Employee> list) { //Your code here return -1; } /** * When the search by Job button is pressed it calls this method * it should search the list of Employees for the job, * returning the index of the first record it finds with * the search string job, or a -1 if it was not found **/ public int findJob(String job, ArrayList<Employee> list) { //Your code here return -1; } }
What to do
- Try to get it running. As it is, it only sorts the information, the search is not yet working.
- Complete the search by name (and or search by job) using a linear search
- Complete the search by name (and or search by job) using a binary search (don't forget to sort first!)
- If you notice the search will only return the first record--try to fill the textArea will all the records that match the search criteria