Grid World 3
<< GridWorld 2 | HomeworkTrailIndex | GridWorld 4 >>
GridWorld Classes and Interfaces
Reference Guide You'll have to use during the exam
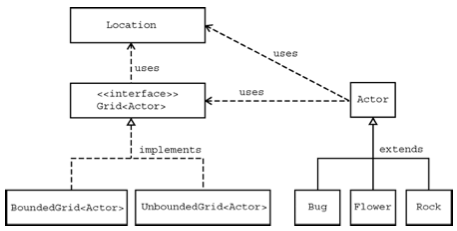
Making new ActorWorlds
import info.gridworld.actor.*; import info.gridworld.grid.*; import java.util.ArrayList; import java.awt.Color; public class CustomBugRunner { public static void main(String[] args) { BoundedGrid gr=new BoundedGrid(5,15); ActorWorld world=new ActorWorld(gr); world.add(new Location(4,0), new Bug() ); ArrayList<Location> occupied = gr.getOccupiedLocations(); for (Location loc:occupied) { Actor a=(Actor)gr.get(loc); a.setDirection(Location.EAST); a.setColor(Color.GREEN); } world.show(); } }
Review Exercises
- Set 3, Nos. 1-5, page 18
The API for the Location
class is in Appendix B. Assume the following statements when answering the following questions.
Location loc1 = new Location(4, 3); Location loc2 = new Location(3, 4);
- How would you access the row value for
loc1
? - What is the value of
b
after the following statement is executed?
- How would you access the row value for
boolean b = loc1.equals(loc2);
- What is the value of
loc3
after the following statement is executed?
- What is the value of
Location loc3 = loc2.getAdjacentLocation(Location.SOUTH;
- What is the value of dir after the following statement is executed?
int dir = loc1.getDirectionToward(new Location(6, 5));
- How does the
getAdjacentLocation
method know which adjacent location to return?
- How does the
- Set 4, Nos 1-4, page 21
The API for the Grid
interface is in Appendix B.
- How can you obtain a count of the objects in a grid? How can you obtain a count of the empty locations in a bounded grid?
- How can you check if location (10,10) is in a grid?
- Grid contains method declarations, but no code is supplied in the methods. Why? Where can you find the implementations of these methods?
- All methods that return multiple objects return them in an ArrayList. Do you think it would be a better design to return the objects in an array? Explain your answer.
- Set 5, Nos. 1-5, page 22
The API for the Actor
class is in Appendix B.
- Name three properties of every actor.
- When an actor is constructed, what is its direction and color?
- Why do you think that the Actor class was created as a class instead of an interface?
- Can an actor put itself into a grid twice without first removing itself? Can an actor remove itself from a grid twice? Can an actor be placed into a grid, remove itself, and then put itself back? Try it out. What happens?
- How can an actor turn 90 degrees to the right?
- Set 6, Nos. 1-11, page 25
The source code for the Bug
class is in Appendix C.
- Which statement(s) in the canMove method ensures that a bug does not try to move out of its grid?
- Which statement(s) in the canMove method determines that a bug will not walk into a rock?
- Which methods of the Grid interface are invoked by the canMove method and why?
- Which method of the Location class is invoked by the canMove method and why?
- Which methods inherited from the Actor class are invoked in the canMove method?
- What happens in the move method when the location immediately in front of the bug is out of the grid?
- Is the variable loc needed in the move method, or could it be avoided by calling getLocation() multiple times?
- Why do you think the flowers that are dropped by a bug have the same color as the bug?
- When a bug removes itself from the grid, will it place a flower into its previous location?
- Which statement(s) in the move method places the flower into the grid at the bug’s previous location?
- If a bug needs to turn 180 degrees, how many times should it call the turn method?
- Group Activity: Jumper, Nos. 1-4, page 26
- Specify: Each group creates a class called Jumper. This actor can move forward two cells in each move. It “jumps” over rocks and flowers. It does not leave anything behind it when it jumps. in the small groups, discuss and clarify the details of the problem:
- What will a jumper do if the location in front of it is empty, but the location two cells in front contains a flower or a rock?
- What will a jumper do if the location two cells in front of the jumper is out of the grid?
- What will a jumper do if it is facing an edge of the grid?
- What will a jumper do if another actor (not a flower or a rock) is in the cell that is two cells in front of the jumper?
- What will a jumper do if it encounters another jumper in its path?
- Are there any other tests the jumper needs to make?
- Design: Groups address important design decisions to solve the problem:
- Which class should
Jumper
extend? - Is there an existing class that is similar to the Jumper class?
- Should there be a constructor? If yes, what parameters should be specified for the constructor?
- Which methods should be overridden?
- What methods, if any, should be added?
- What is the plan for testing the class?
- Which class should
- Code: Implement the
Jumper
andJumperRunner
classes. - Test: Carry out the test plan to verify that the
Jumper
class meets the
- Specify: Each group creates a class called Jumper. This actor can move forward two cells in each move. It “jumps” over rocks and flowers. It does not leave anything behind it when it jumps. in the small groups, discuss and clarify the details of the problem:
specification.
Jumper.java
import info.gridworld.actor.Actor; import info.gridworld.actor.Flower; import info.gridworld.actor.Rock; import info.gridworld.grid.Grid; import info.gridworld.grid.Location; import java.awt.Color; /** * A Jumper is an actor that will jump over Rocks and Flowers * Faced with the edge it will scroll "Pac-Man" style. *****/
JumperRunner.java
import info.gridworld.actor.*; import info.gridworld.grid.*; /** * This class runs a world that contains a jumper, a bug, a flower, and a * rock added at random locations. */ public class JumperRunner { public static void main(String[] args) { ActorWorld world = new ActorWorld(); world.add(new Location (2,3), new Jumper() ); world.add(new Location (1,3), new Rock ()); world.add(new Bug()); world.add(new Flower()); world.show(); } }
Programming Exercises
Part3Set4.java
import info.gridworld.grid.*; import java.util.ArrayList; /** * This class runs a world that helps test out answers to Set 4. * */ public class Part3Set4 { public static void main(String[] args) { BoundedGrid gr=new BoundedGrid(10,10); Location loc1 = new Location(4,3); Location loc2 = new Location(1,1); gr.put(loc1, "keys"); gr.put(loc2, "coins"); gr.put(new Location(2,1), 7); ArrayList stuff=gr.getOccupiedLocations(); System.out.println(stuff); System.out.println(stuff.size()); System.out.println( (String)gr.get(loc1) ); System.out.println( gr.get(new Location (2,1) ) ); System.out.println("There are "+gr.getOccupiedLocations().size()+" things in the grid"); } }
Part3Set6Number9.java
import info.gridworld.actor.*; import info.gridworld.grid.*; public class Part3Set6Number9 { public static void main(String[] args) { ActorWorld world = new ActorWorld(); //ActorWorld is not Testable.. see page 27 Bug b = new Bug(); world.add(new Location(9,9), b); world.show(); b.removeSelfFromGrid(); } }
Labs
Quarantine
Write a main method that populates a 20 by 20 ActorWorld
with 10 randomly placed Bugs. Then surround the Bugs with rocks.
import info.gridworld.actor.*; import info.gridworld.grid.*; import java.util.ArrayList; import java.awt.Color; public class Quarantine { public static void main(String[] args) { } }
War
Make a main method that constructs a 40 by 60 ActorWorld with 20 bugs on the left and 20 bugs on the right, facing each other. This will be similar to Bug Battle on YouTube. The left bugs should be red, the right bugs should be green.