Loading Image Files From Your Jar
<< Removing Windoze Flicker | Applets | Keyboard Listener Demo >>
You can download the two files background.jpg and butterfly.gif and when you make your project in your IDE, drag them to your project folder. Eventually they will be part of your java archive or "Jar" file. The method to look at here is loadImageFromJar
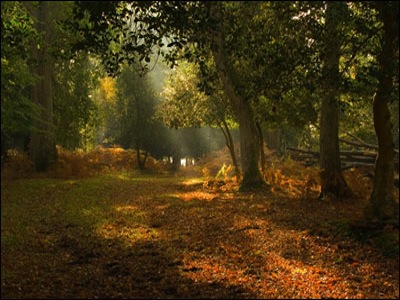

MovingPictures.java
import java.applet.Applet; import java.awt.Color; import java.awt.Font; import java.awt.Graphics; import java.awt.Image; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.IOException; import javax.imageio.ImageIO; import javax.swing.Timer; public class MovingPictures extends Applet implements ActionListener { public static final int WIDTH=400,HEIGHT=300; private int x, y; private Timer timer; private Image imgBuffer; private Graphics gBuffer; private Font font; private Image background, butterfly; /** * imgBuffer is the "off screen" graphics area * gBuffer is imgBuffer's graphics object * which we need to draw stuff on the image */ public void init() { background=loadImageFromJar("background.jpg"); butterfly=loadImageFromJar("butterfly.gif"); imgBuffer = createImage(WIDTH,HEIGHT); gBuffer = imgBuffer.getGraphics(); //Here we make a timer event every 60 millisecs timer = new Timer(60,this); timer.start(); x = this.getWidth()/2; y = this.getHeight()/2; font = new Font("Helvetica", Font.BOLD, 18); } /** * Your IDE can place resources like images and sounds * inside the same jar as your code, so here is an * example of how to load an image * @param fileName * @return an Image */ public Image loadImageFromJar(String fileName) { Image img=null; try { img = getImage( getClass().getResource("/"+fileName)); } catch (NullPointerException e) { //e.printStackTrace(); } return img; } public void paint(Graphics g) { // draw components off screen on gBufffer if (background!=null){ gBuffer.drawImage(background, 0, 0, WIDTH, HEIGHT, null); } else { gBuffer.setColor(Color.WHITE); gBuffer.fillRect(0, 0, WIDTH, HEIGHT); gBuffer.setColor(Color.RED); gBuffer.drawString("background image not found", 20, 60); } if (butterfly!=null){ gBuffer.drawImage(butterfly, x, y, null); } else { gBuffer.setColor(Color.CYAN); gBuffer.fillOval(x, y, 50, 50); gBuffer.setColor(Color.RED); gBuffer.drawString("butterfly image not found", 20, 80); } gBuffer.setColor(Color.CYAN); gBuffer.setFont(font); gBuffer.drawString("X = "+x, 20, 40); // now we quickly swap the screen image with the one we made g.drawImage(imgBuffer,0,0,this); } /** * update is only needed for Microsoft Windows * to remove the famous "flicker problem" */ public void update(Graphics g) { paint(g); } /** * Here we implement the ActionLister interface. * Our time produced an action event over the * passage of time. So to animate our applet * we change the horizontal location of out Oval. */ @Override public void actionPerformed(ActionEvent e) { x+=3; x=x%(WIDTH-50); repaint(); } }
Exporting your Applet to work on a web page
After you make your jar file ("Export" in Eclipse or "Make Jar" in BlueJ), you need a HTML or web page that looks like:
<html> <head> <title>MovingPictures Applet</title> </head> <body> <h1>Moving Pictures Applet</h1> <hr> <applet code="MovingPictures.class" width=400 height=300 archive="MovingPictures.jar" alt="Your browser understands the <APPLET> tag but isn't running the applet, for some reason." > Your browser is ignoring the <APPLET> tag! </applet> <hr> </body> </html>