Questions 20
<< ConsoleApplet | OtherProjectsTrailIndex | Basic Pong >>
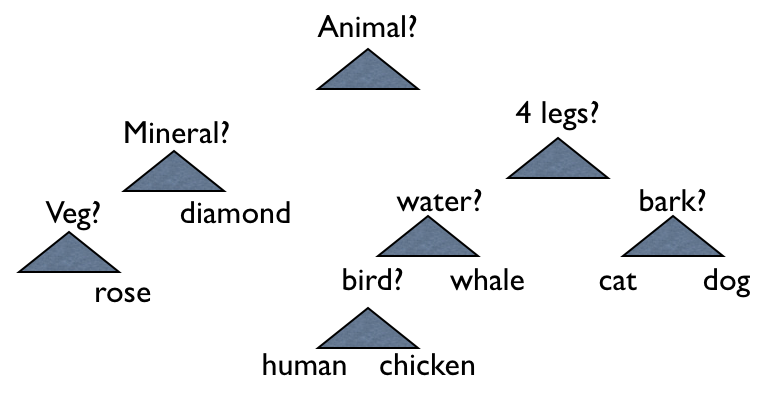
questions.txt
-1 1 6 Is it an animal? 0 3 4 Is it a mineral? 10 -1 -1 Is it a whale? 1 -1 5 Is it a vegetable? 1 -1 -1 Is it a diamond? 3 -1 -1 Is it a rose 0 10 8 Does it have 4 legs? 8 -1 -1 Is it a dog? 6 9 7 Does it bark? 8 -1 -1 Is it a cat? 6 12 2 Does it spend most of its time in the water? 12 -1 -1 Is it a human? 10 11 13 is it a bird? 12 -1 -1 Is it a chicken?
Quesiton.java
public class Question { private int parent; private int noLink; private int yesLink; private String quest; public Question(){ setParent(-1); setNoLink(-1); setYesLink(-1); setQuest(""); } public Question(String[] s){ setParent(Integer.parseInt(s[0])); setNoLink(Integer.parseInt(s[1])); setYesLink(Integer.parseInt(s[2])); setQuest(s[3]); } public void setParent(int parent) { this.parent = parent; } public int getParent() { return parent; } public void setNoLink(int noLink) { this.noLink = noLink; } public int getNoLink() { return noLink; } public void setYesLink(int yesLink) { this.yesLink = yesLink; } public int getYesLink() { return yesLink; } public void setQuest(String quest) { this.quest = quest; } public String getQuest() { return quest; } public String toString(){ return parent+"\t"+noLink+"\t"+yesLink+"\t"+quest; } public String lastWord(){ String lastThing=quest.substring(quest.lastIndexOf(" "),quest.lastIndexOf("?")); //lastThing=lastThing.substring(0, lastThing.length()-1); return lastThing; } }
Questions20.java (The Applet version, which is not normally allowed to write files)
import java.applet.Applet; import java.awt.*; import java.awt.event.*; import java.io.*; import java.net.URL; import java.util.*; import javax.swing.*; @SuppressWarnings("serial") public class Questions20 extends Applet implements ActionListener { private ArrayList<Question> questions; private Question currentQuestion; private int count; private TextArea t; private Button yesButton,noButton, restartButton; public void init(){ //Init the user interface objects t=new TextArea(); t.setFont(new Font("Helvetica", Font.BOLD,20)); t.setForeground(new Color(251,182,0)); t.setBackground(new Color(44,23,0)); t.setEditable(false); yesButton=new Button("Yes"); yesButton.addActionListener(this); noButton=new Button("No"); noButton.addActionListener(this); restartButton=new Button("Start Over"); restartButton.addActionListener(this); //Add objects to Applet this.setLayout(new BorderLayout()); this.add(t, BorderLayout.CENTER); Panel buttonPanel=new Panel(new FlowLayout()); buttonPanel.add(yesButton); buttonPanel.add(noButton); buttonPanel.add(restartButton); this.add(buttonPanel, BorderLayout.NORTH); startOver(); } private void startOver() { questions=readQuestionFile(); currentQuestion=questions.get(0); count=1; t.append("Twenty Questions!\n1. "+currentQuestion.getQuest()); } @Override public void actionPerformed(ActionEvent e) { Object buttonPressed=e.getSource(); if (buttonPressed==yesButton){ if (currentQuestion.getYesLink() <0){ //computer guessed it t.append(" YES !!!! I Guessed it with "+count+" questions!!\n"); }else{ currentQuestion=questions.get(currentQuestion.getYesLink()); count++; t.append(" YES\n"+count+". "+currentQuestion.getQuest()); } } else if (buttonPressed==noButton){ if (currentQuestion.getNoLink()<0){ //Make a new Question t.append(" NO\nAfter "+count+" questions, I have no idea! What is it?"); getInfoDialog(currentQuestion.lastWord()); }else{ currentQuestion=questions.get(currentQuestion.getNoLink()); count++; t.append(" NO\n"+count+". "+currentQuestion.getQuest()); } } if (buttonPressed==restartButton) { t.setText(""); startOver(); } } public void writeQuestionFile(ArrayList<Question> q){ //PrintWriter outFile; try { //outFile = new PrintWriter("questions.txt"); File f=new File("questions.txt"); BufferedWriter out = new BufferedWriter(new FileWriter(f,false)); for (int i=0; i<q.size(); i++){ out.write(q.get(i).toString()+"\r\n"); } out.close(); } catch (Exception e) { e.printStackTrace(); } } public ArrayList<Question> readQuestionFile() { ArrayList<Question> result=new ArrayList<Question>(); try{ InputStream is=this.getClass().getResourceAsStream("questions.txt"); Scanner in = new Scanner(is); String line = null; while (in.hasNextLine()) { line = in.nextLine(); result.add(new Question(line.split("\t"))); } in.close(); } catch(Exception e){ e.printStackTrace(); } return result; } public void getInfoDialog(String lastThing){ JTextField thing=new JTextField(); final JComponent[] newQ = new JComponent[] { new JLabel("I have no idea! What is it?"), thing }; JOptionPane.showMessageDialog(this, newQ, "New Object Found!", JOptionPane.PLAIN_MESSAGE); thing.requestFocusInWindow(); try{ t.append(" A "+thing.getText()); getInfoDialog(lastThing, thing.getText()); }catch( Exception exception){ t.append("\nI can't deal with this. Try again\n"); } } public void getInfoDialog(String lastThing, String thing){ JTextField q=new JTextField(); final JComponent[] newQ = new JComponent[] { new JLabel("Type a yes/no question that would distinguish between a "+lastThing+" and a "+thing), q }; q.requestFocus(); JOptionPane.showMessageDialog(this, newQ, "Tell me about It", JOptionPane.PLAIN_MESSAGE); q.requestFocus(); try{ confirmDialog(thing, q.getText()); }catch( Exception exception){ t.append("\nI can't deal with this. Try again\n"); } } public void confirmDialog(String thing, String quest){ final JComponent[] newQ = new JComponent[] { new JLabel("And for a "+thing+", what is the answer to the question "), new JLabel("\""+quest+"\"") , new JLabel("(Press CANCEL if you are unsure)") }; int resp=JOptionPane.showConfirmDialog(this, newQ, "Tell me about It", JOptionPane.YES_NO_CANCEL_OPTION); try{ if (resp==JOptionPane.CANCEL_OPTION) { t.append("\nDatabase unchanged\n"); return; } String newQuestAnswer="y"; if (resp==JOptionPane.YES_OPTION){ t.append("\nAdding the question \""+quest+"\" \n(\"Yes\" for a "+thing+")\n"); } else if (resp==JOptionPane.NO_OPTION){ t.append("\nAdding the question \""+quest+"\" \n(\"No\" for a "+thing+")\n"); newQuestAnswer="n"; } update(questions, currentQuestion, thing, quest, newQuestAnswer); }catch( Exception exception){ t.append("\nI can't deal with this. Try again\n"); } } private void update(ArrayList<Question> list, Question currentQuestion, String thing, String newQuest, String newQuestAnswer) { int n=list.size(); // New Question's index //Now have the previous question point to the question: Question currentQuestionRoot=list.get(currentQuestion.getParent()); if (list.indexOf(currentQuestion)==currentQuestionRoot.getYesLink()){ currentQuestionRoot.setYesLink(n); } else { currentQuestionRoot.setNoLink(n); } //Make New stuff Question q=new Question(); q.setQuest(newQuest); q.setParent(currentQuestion.getParent()); //New Question is the parent of the thing it wasn't currentQuestion.setParent(n); newQuestAnswer=newQuestAnswer.toLowerCase(); if (newQuestAnswer.startsWith("y")){ q.setYesLink(n+1); q.setNoLink(list.indexOf(currentQuestion)); } else { q.setYesLink(list.indexOf(currentQuestion)); q.setNoLink(n+1); } Question t=new Question(); t.setParent(n); t.setNoLink(-1); t.setYesLink(-1); t.setQuest("Is it a "+thing+"?"); list.add(q); list.add(t); writeQuestionFile(list); } }
Questions20App.java (This is the GUI Application which can update the questions.txt file)
import java.awt.*; import java.awt.event.*; import java.io.*; import java.util.*; import javax.swing.*; @SuppressWarnings("serial") public class Questions20App extends JFrame implements ActionListener { private ArrayList<Question> questions; private Question currentQuestion; private int count; private TextArea t; private Button yesButton,noButton, restartButton; public Questions20App() { //Init the user interface objects t=new TextArea(); t.setFont(new Font("Helvetica", Font.BOLD,20)); t.setForeground(new Color(251,182,0)); t.setBackground(new Color(44,23,0)); t.setEditable(false); yesButton=new Button("Yes"); yesButton.addActionListener(this); noButton=new Button("No"); noButton.addActionListener(this); restartButton=new Button("Start Over"); restartButton.addActionListener(this); //Add objects to Applet this.setLayout(new BorderLayout()); this.add(t, BorderLayout.CENTER); Panel buttonPanel=new Panel(new FlowLayout()); buttonPanel.add(yesButton); buttonPanel.add(noButton); buttonPanel.add(restartButton); this.add(buttonPanel, BorderLayout.NORTH); startOver(); setSize(800,600); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } private void startOver() { questions=readQuestionFile(); currentQuestion=questions.get(0); count=1; t.append("Twenty Questions!\n1. "+currentQuestion.getQuest()); } @Override public void actionPerformed(ActionEvent e) { Object buttonPressed=e.getSource(); if (buttonPressed==yesButton){ if (currentQuestion.getYesLink() <0){ //computer guessed it t.append(" YES !!!! I Guessed it with "+count+" questions!!\n"); }else{ currentQuestion=questions.get(currentQuestion.getYesLink()); count++; t.append(" YES\n"+count+". "+currentQuestion.getQuest()); } } else if (buttonPressed==noButton){ if (currentQuestion.getNoLink()<0){ //Make a new Question t.append(" NO\nAfter "+count+" questions, I have no idea! What is it?"); getInfoDialog(currentQuestion.lastWord()); }else{ currentQuestion=questions.get(currentQuestion.getNoLink()); count++; t.append(" NO\n"+count+". "+currentQuestion.getQuest()); } } if (buttonPressed==restartButton) { t.setText(""); startOver(); } } public void writeQuestionFile(ArrayList<Question> q){ //PrintWriter outFile; try { //outFile = new PrintWriter("questions.txt"); File f=new File("questions.txt"); BufferedWriter out = new BufferedWriter(new FileWriter(f,false)); for (int i=0; i<q.size(); i++){ out.write(q.get(i).toString()+"\r\n"); } out.close(); } catch (Exception e) { e.printStackTrace(); } } public ArrayList<Question> readQuestionFile() { ArrayList<Question> result=new ArrayList<Question>(); try{ InputStream is=this.getClass().getResourceAsStream("questions.txt"); Scanner in = new Scanner(is); String line = null; while (in.hasNextLine()) { line = in.nextLine(); result.add(new Question(line.split("\t"))); } in.close(); } catch(Exception e){ e.printStackTrace(); } return result; } public void getInfoDialog(String lastThing){ JTextField thing=new JTextField(); final JComponent[] newQ = new JComponent[] { new JLabel("I have no idea! What is it?"), thing }; JOptionPane.showMessageDialog(this, newQ, "New Object Found!", JOptionPane.PLAIN_MESSAGE); thing.requestFocusInWindow(); try{ t.append(" A "+thing.getText()); getInfoDialog(lastThing, thing.getText()); }catch( Exception exception){ t.append("\nI can't deal with this. Try again\n"); } } public void getInfoDialog(String lastThing, String thing){ JTextField q=new JTextField(); final JComponent[] newQ = new JComponent[] { new JLabel("Type a yes/no question that would distinguish between a "+lastThing+" and a "+thing), q }; q.requestFocus(); JOptionPane.showMessageDialog(this, newQ, "Tell me about It", JOptionPane.PLAIN_MESSAGE); q.requestFocus(); try{ confirmDialog(thing, q.getText()); }catch( Exception exception){ t.append("\nI can't deal with this. Try again\n"); } } public void confirmDialog(String thing, String quest){ final JComponent[] newQ = new JComponent[] { new JLabel("And for a "+thing+", what is the answer to the question "), new JLabel("\""+quest+"\"") , new JLabel("(Press CANCEL if you are unsure)") }; int resp=JOptionPane.showConfirmDialog(this, newQ, "Tell me about It", JOptionPane.YES_NO_CANCEL_OPTION); try{ if (resp==JOptionPane.CANCEL_OPTION) { t.append("\nDatabase unchanged\n"); return; } String newQuestAnswer="y"; if (resp==JOptionPane.YES_OPTION){ t.append("\nAdding the question \""+quest+"\" \n(\"Yes\" for a "+thing+")\n"); } else if (resp==JOptionPane.NO_OPTION){ t.append("\nAdding the question \""+quest+"\" \n(\"No\" for a "+thing+")\n"); newQuestAnswer="n"; } update(questions, currentQuestion, thing, quest, newQuestAnswer); }catch( Exception exception){ t.append("\nI can't deal with this. Try again\n"); } } private void update(ArrayList<Question> list, Question currentQuestion, String thing, String newQuest, String newQuestAnswer) { int n=list.size(); // New Question's index //Now have the previous question point to the question: Question currentQuestionRoot=list.get(currentQuestion.getParent()); if (list.indexOf(currentQuestion)==currentQuestionRoot.getYesLink()){ currentQuestionRoot.setYesLink(n); } else { currentQuestionRoot.setNoLink(n); } //Make New stuff Question q=new Question(); q.setQuest(newQuest); q.setParent(currentQuestion.getParent()); //New Question is the parent of the thing it wasn't currentQuestion.setParent(n); newQuestAnswer=newQuestAnswer.toLowerCase(); if (newQuestAnswer.startsWith("y")){ q.setYesLink(n+1); q.setNoLink(list.indexOf(currentQuestion)); } else { q.setYesLink(list.indexOf(currentQuestion)); q.setNoLink(n+1); } Question t=new Question(); t.setParent(n); t.setNoLink(-1); t.setYesLink(-1); t.setQuest("Is it a "+thing+"?"); list.add(q); list.add(t); writeQuestionFile(list); } public static void main(String[] args) { Questions20App app= new Questions20App(); app.setVisible(true); } }