Rock Hound
Write a subclass of the Actor class called RockHound. The RockHound loves to to move toward any Rock in the grid. You will need to write two methods, getRockLocation and act.
Part (a): Write the method getRockLocation. It returns either a valid Location in the grid gr that has a Rock in it, or else null if there are no Rocks in the grid.
Part (b): Complete the method act. If there is a Rock in the grid, it turns toward the Rock. If the the adjacent location toward the Rock is valid and empty, it moves there. If there is no Rock in the grid, it behaves like an Actor. You may presume the method getRockLocation works properly, regardless of your answer to part (a).
RockHound.gif
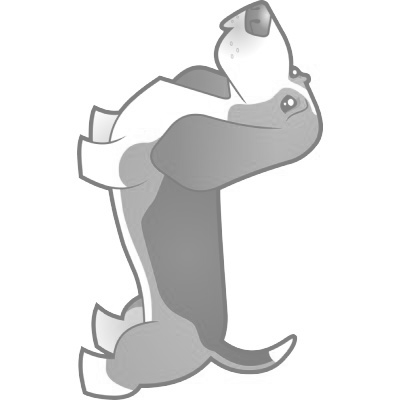
RockHoundTester.java
import info.gridworld.actor.*; import info.gridworld.grid.*; import java.awt.Color; public class RockHoundTester { public static void main(String[] args) { ActorWorld world = new ActorWorld(); world.add(new Location(7, 8), new Rock()); world.add(new Location(3, 3), new Rock()); world.add( new RockHound()); world.add(new Location(8, 0), new Bug()); world.show(); } }
RockHound.java
import java.util.ArrayList; import info.gridworld.actor.*; import info.gridworld.grid.*; public class RockHound extends Actor { public Location getRockLocation(){ //part (a) } public void act(){ //part (b) } }