Rock Paper Scissors
<< Simon Game | LabTrailIndex | Joust >>
The classic Game.. each gesture has 1 predator and 1 prey:
- rock smashes scissors
- scissors cuts paper
- paper covers rock
But we can always add the Big Bang Theory version where each gesture can kill two, and be killed by two:
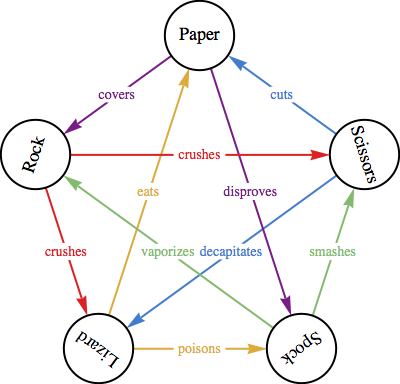
- rock crushes scissors
- rock crushes lizard
- scissors cuts paper
- scissors decapitate lizard
- paper covers rock
- paper disproves spock
- lizard poisons spock
- lizard eats paper
- spock smashes scissors
- spock vaporizes rock
Click Here for a working demonstration
We will make first make the classic game with 3 classes (Rock, Paper, Scissors). Each will implement the Gesture
interface.
Gesture.java
interface Gesture { int beats(Gesture g); String action(Gesture g); }
beats
works so thatrock.beats(paper)
returns-1
(it loses to paper) andscissors.beats(paper)
returns1
(it wins over paper) and ties, returns 0.action
works so thatrock.action(paper)
returns " covered by " andscissors.action(paper)
returns " cuts ". If there is a tie, it should return " ties with "
(once we study Abstract Classes, we may want to make Gesture
an Abstract class so we can add some common code, rather than have each implementation do certain things)
GestureTester.java
import java.util.ArrayList; public class GestureTester { /** * @param args */ public static void main(String[] args) { ArrayList<Gesture> list = new ArrayList<Gesture>(); list.add(new Rock() ); list.add(new Paper() ); list.add(new Scissors() ); // list.add(new Lizard() ); // list.add(new Spock() ); for (int i=0;i<list.size();i++){ for(int j=0;j<list.size();j++) test(list.get(i),list.get(j)); System.out.println(); } } public static void test(Gesture g1, Gesture g2){ System.out.println(g1+g1.action(g2)+g2+", so "+g1+".beats("+g2+") method returns "+g1.beats(g2)); } }
Example output of tester with the normal 3 gestures:
rock ties with rock, so rock.beats(rock) method returns 0 rock covered by paper, so rock.beats(paper) method returns -1 rock crushes scissors, so rock.beats(scissors) method returns 1 paper covers rock, so paper.beats(rock) method returns 1 paper ties with paper, so paper.beats(paper) method returns 0 paper cut by scissors, so paper.beats(scissors) method returns -1 scissors crushed by rock, so scissors.beats(rock) method returns -1 scissors cuts paper, so scissors.beats(paper) method returns 1 scissors ties with scissors, so scissors.beats(scissors) method returns 0
or with all five:
rock ties with rock, so rock.beats(rock) method returns 0 rock covered by paper, so rock.beats(paper) method returns -1 rock crushes scissors, so rock.beats(scissors) method returns 1 rock crushes lizard, so rock.beats(lizard) method returns 1 rock vaporized by spock, so rock.beats(spock) method returns -1 paper covers rock, so paper.beats(rock) method returns 1 paper ties with paper, so paper.beats(paper) method returns 0 paper cut by scissors, so paper.beats(scissors) method returns -1 paper eaten by lizard, so paper.beats(lizard) method returns -1 paper disproves spock, so paper.beats(spock) method returns 1 scissors crushed by rock, so scissors.beats(rock) method returns -1 scissors cuts paper, so scissors.beats(paper) method returns 1 scissors ties with scissors, so scissors.beats(scissors) method returns 0 scissors decapitates lizard, so scissors.beats(lizard) method returns 1 scissors smashed by spock, so scissors.beats(spock) method returns -1 lizard crushed by rock, so lizard.beats(rock) method returns -1 lizard eats paper, so lizard.beats(paper) method returns 1 lizard decapitated by scissors, so lizard.beats(scissors) method returns -1 lizard ties with lizard, so lizard.beats(lizard) method returns 0 lizard poisons spock, so lizard.beats(spock) method returns 1 spock vaporizes rock, so spock.beats(rock) method returns 1 spock disproved by paper, so spock.beats(paper) method returns -1 spock smashes scissors, so spock.beats(scissors) method returns 1 spock poisoned by lizard, so spock.beats(lizard) method returns -1 spock ties with spock, so spock.beats(spock) method returns 0
To get you started, here is how I made the Rock class:
Rock.java
public class Rock implements Gesture { public int beats(Gesture g) { if (g instanceof Scissors) return 1; if (g instanceof Paper) return -1; return 0; } public String action(Gesture g) { if (this.beats(g)>0){ if (g instanceof Scissors) return " crushes "; } if (this.beats(g)<0){ if (g instanceof Paper) return " covered by "; } return " ties with "; } public String toString() { String className = this.getClass().toString().toLowerCase(); return className.substring(className.indexOf(" ")+1); } }
When you are don, I hope you notice that there is a lot of repetition writing these particular implementations of the Gesture interface. Next (Chapter 10 stuff) we can see how to make them subclasses of a super class as we move to a graphical Applet like the demo. You can see the code here