Alexis Final Project
<< Alexander's Final Project | OldProjectsTrailIndex | Cameron's Final Project >>
Click here for a working demonstration
Files
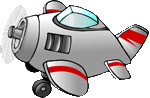
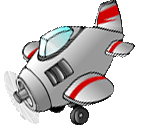
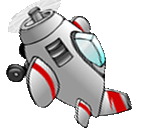



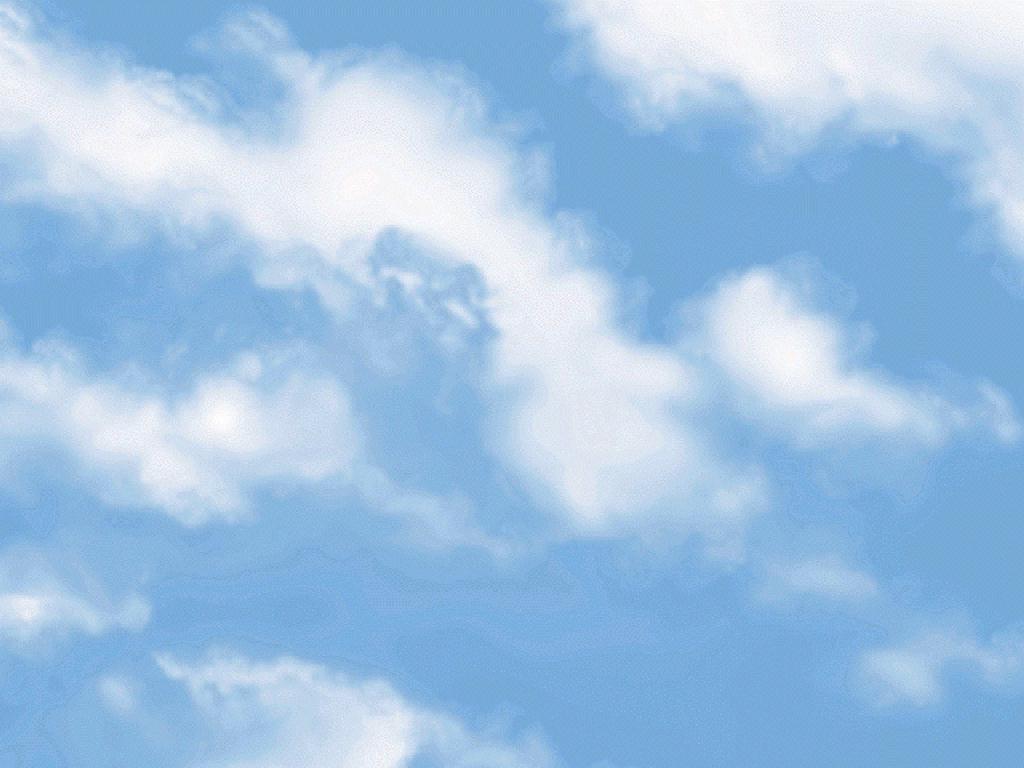
Bullet.java
import java.awt.Graphics; import java.awt.event.ActionEvent; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import javax.imageio.ImageIO; import javax.swing.Timer; public class Bullet { private int x; private int y; boolean left; private BufferedImage img; // see http://download.oracle.com/javase/tutorial/2d/images/loadimage.html public Bullet(String graphicFileName, int x, int y){ this.x=x; this.y=y; left=false; this.img = null; try { img = ImageIO.read(new File(graphicFileName)); } catch (IOException e) { } } public int getX() {return x;} public int getY() {return y;} /** * if x changes, we might need to switch the direction our monster is facing * @param x */ public void setX(int x){ left=true; if (x>this.x){ left=false; } this.x=x; } public void setY(int y){ this.y=y;} // see http://download.oracle.com/javase/tutorial/2d/images/drawimage.html /** * this is a basic way of drawing the whole img, as it is */ public void draw(Graphics g){ g.drawImage(img, x, y, null); } public void actionPerformed(ActionEvent e) { } }
Plane.java
import java.awt.Graphics; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import javax.imageio.ImageIO; public class Plane { private int x; private int y; boolean left; private int lives; private BufferedImage img; // see http://download.oracle.com/javase/tutorial/2d/images/loadimage.html public Plane(String graphicFileName, int x, int y){ this.x=x; this.y=y; lives=3; left=false; this.img = null; try { img = ImageIO.read(new File(graphicFileName)); } catch (IOException e) { } } public int getX() {return x;} public int getY() {return y;} /** * if x changes, we might need to switch the direction our monster is facing * @param x */ public void setX(int x){ left=true; if (x>this.x){ left=false; } this.x=x; } public void setY(int y){ this.y=y;} // see http://download.oracle.com/javase/tutorial/2d/images/drawimage.html /** * this is a basic way of drawing the whole img, as it is */ public void draw(Graphics g){ g.drawImage(img, x, y, null); } }
RedBird.java
import java.awt.Graphics; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import javax.imageio.ImageIO; import java.util.Random; public class RedBird { private int x; private int y; boolean left; private BufferedImage img; // see http://download.oracle.com/javase/tutorial/2d/images/loadimage.html public RedBird(String graphicFileName){ x=0; Random generator = new Random(); y= generator.nextInt(540); left=false; this.img = null; try { img = ImageIO.read(new File(graphicFileName)); } catch (IOException e) { } } public int getX() {return x;} public int getY() {return y;} /** * if x changes, we might need to switch the direction our monster is facing * @param x */ public void setX(int x){ left=true; if (x>this.x){ left=false; } this.x=x; } public void setY(int y){ this.y=y;} // see http://download.oracle.com/javase/tutorial/2d/images/drawimage.html /** * this is a basic way of drawing the whole img, as it is */ public void draw(Graphics g){ g.drawImage(img, x, y, null); } public void dead(){ this.x= -90; this.y= -60; } }
driller.java
import java.awt.Graphics; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import javax.imageio.ImageIO; import java.util.Random; public class Driller { private int x; private int y; boolean left; private BufferedImage img; // see http://download.oracle.com/javase/tutorial/2d/images/loadimage.html public Driller(String graphicFileName){ x=0; Random generator = new Random(); y= generator.nextInt(540); left=false; this.img = null; try { img = ImageIO.read(new File(graphicFileName)); } catch (IOException e) { } } public int getX() {return x;} public int getY() {return y;} /** * if x changes, we might need to switch the direction our monster is facing * @param x */ public void setX(int x){ left=true; if (x>this.x){ left=false; } this.x=x; } public void setY(int y){ this.y=y;} // see http://download.oracle.com/javase/tutorial/2d/images/drawimage.html /** * this is a basic way of drawing the whole img, as it is */ public void draw(Graphics g){ g.drawImage(img, x, y, null); } public void dead(){ this.x= -90; this.y= -60; } }
Background.java
import java.awt.Graphics; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import javax.imageio.ImageIO; public class Background { private int x; private int y; private BufferedImage img; public Background(String graphicFileName, int x, int y){ this.x=x; this.y=y; this.img = null; try { img = ImageIO.read(new File(graphicFileName)); } catch (IOException e) { } } public void draw(Graphics g){ g.drawImage(img, x, y, null); } }
Sound.java
import java.net.URL; import java.applet.*; public class Sound { private String fileName; private AudioClip player; public Sound(String file){ fileName=file; player=null; } public void play() { try { URL soundToPlay = getClass().getResource(fileName); player = Applet.newAudioClip(soundToPlay); player.play(); } catch (Exception e) {} } public void loop() { try { URL soundToPlay = getClass().getResource(fileName); player = Applet.newAudioClip(soundToPlay); player.loop(); } catch (Exception e) {} } }
PlaneFrenzy.java
import java.applet.Applet; import java.awt.Graphics; import java.awt.Image; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; import java.util.Random; import javax.swing.Timer; import sun.audio.*; /** * Demonstrates an 3D Object like a cube * and makes a "First-person" point-of view * using the arrow keys to move up and down, left and right * size of applet should be 800 x 600 */ @SuppressWarnings("serial") public class PlaneFrenzy extends Applet implements KeyListener, ActionListener { public static final int amount=5; //amount of change each arrow will change private Plane plane; private RedBird redB; private Driller driller; private Bullet bullet; private Background bg; private Timer score; private Timer bulletMovement; private Timer RBMovement; private Timer RBSpawner; private Timer DMovement; private Timer DSpawner; private Image virtualMem; private Graphics gBuffer; private Random RBSChance; private Random DChance; private Sound bgm; private int appH; private int appW; private int userScore; private int kills; private int RBSRate; private int DRate; private boolean DKilled; public void init(){ kills = 0; RBSRate = 500; DRate = 500; DKilled = false; plane = new Plane("userplane.gif", 650, 300); bg = new Background("background.jpg", 0, 0); score = new Timer(1000, this); bulletMovement = new Timer(1, this); RBMovement = new Timer(6, this); RBSpawner = new Timer(RBSRate, this); DMovement = new Timer(5, this); DSpawner = new Timer(DRate, this); bgm = new Sound("bgm.wav"); bgm.loop(); RBSpawner.start(); score.start(); RBSChance = new Random(); DChance = new Random(); appH = getHeight(); appW = getWidth(); virtualMem = createImage(appW, appH); gBuffer = virtualMem.getGraphics(); this.addKeyListener(this); } public void paint(Graphics g){ virtualMem = createImage(appW, appH); gBuffer = virtualMem.getGraphics(); bg.draw(gBuffer); if(bulletMovement.isRunning()){ bullet.draw(gBuffer); } if(RBMovement.isRunning()){ redB.draw(gBuffer); } if(DMovement.isRunning()){ driller.draw(gBuffer); } plane.draw(gBuffer); gBuffer.drawString("YOU HAVE SURVIVED FOR "+userScore+" SECONDS AND HAVE KILLED "+kills+" ENEMIES", 20, 580); gBuffer.drawString(Integer.toString(RBSRate), 20, 20); g.drawImage(virtualMem,0,0,this); } public void update(Graphics g) { paint(g); //get rid of flicker with this method } @Override // shift==16 spacebar==32 public void keyPressed(KeyEvent e) { int keyCode=e.getKeyCode(); if(keyCode==38){ //up plane.setY(plane.getY()-amount); plane = new Plane("userplane_up.gif", plane.getX(), plane.getY()); } else if (keyCode==40){ //down plane.setY(plane.getY()+amount); plane = new Plane("userplane_down.gif", plane.getX(), plane.getY()); } else if (keyCode==37){ //left plane.setX(plane.getX()-amount); plane = new Plane("userplane.gif", plane.getX(), plane.getY()); } else if (keyCode==39){ //right plane.setX(plane.getX()+amount*2); plane = new Plane("userplane.gif", plane.getX(), plane.getY()); } else if (keyCode==65){ //left up plane.setX(plane.getX()-amount); plane.setY(plane.getY()-amount); plane = new Plane("userplane_up.gif", plane.getX(), plane.getY()); } else if (keyCode==68){ //right up plane.setX(plane.getX()-amount); plane.setY(plane.getY()+amount); plane = new Plane("userplane_down.gif", plane.getX(), plane.getY()); } else if (keyCode==32){ //fire bullet = new Bullet("bullet.gif", plane.getX(), plane.getY()+7); bulletMovement.start(); } else if (keyCode==75){ //kills cheat kills++; } else if (keyCode==83){ //seconds cheat userScore+= 100; } repaint(); } @Override public void keyReleased(KeyEvent e) {} @Override public void keyTyped(KeyEvent e) {} /** * Timer Section */ @Override public void actionPerformed(ActionEvent e) { Object source = e.getSource(); if(DKilled == false){ if(kills >= 10){ DSpawner.start(); DKilled = true; } } if(source == score) userScore++; //BULLET MOVEMENT else if(source == bulletMovement){ bullet.setX(bullet.getX()-5); if(RBMovement.isRunning()){ if(bullet.getX()>redB.getX()-2 && bullet.getX()<redB.getX()+45 && bullet.getY()>redB.getY()-10 && bullet.getY()<redB.getY()+30){ bulletMovement.stop(); RBMovement.stop(); redB.dead(); kills += 1; RBSRate -= 2; RBSpawner = new Timer(RBSRate, this); RBSpawner.start(); } } if(DMovement.isRunning()){ if(bullet.getX()>driller.getX()-2 && bullet.getX()<driller.getX()+45 && bullet.getY()>driller.getY()-10 && bullet.getY()<driller.getY()+30){ bulletMovement.stop(); DMovement.stop(); driller.dead(); kills += 1; //if statement for next enemy add here DRate -= 2; DSpawner = new Timer(RBSRate, this); DSpawner.start(); } } } //END OF BULLET else if(source == RBMovement){ if(redB.getX() == 800){ redB.dead(); RBMovement.stop(); RBSRate -= 4; RBSpawner = new Timer(RBSRate, this); RBSpawner.start(); } else redB.setX(redB.getX()+1); } else if(source == RBSpawner){ int i = RBSChance.nextInt(4); if(i == 1){ redB = new RedBird("redb.gif"); RBMovement.start(); RBSpawner.stop(); } } else if(source == DSpawner){ int d = DChance.nextInt(4); if(d==1){ driller = new Driller("driller.gif"); DMovement.start(); DSpawner.stop(); } } else if(source == DMovement){ if(driller.getX() > 799){ driller.dead(); DMovement.stop(); DRate -= 4; DSpawner = new Timer(DRate, this); DSpawner.start(); } if(driller.getX()>300)driller.setX(driller.getX()+8); else driller.setX(driller.getX()+1); } repaint(); } }