Camerons Final Project
<< Alexi's Final Project | OldProjectsTrailIndex | Ethan's Final Project >>
Click here to play the latest posted version of the game. Changes may be made at a later date.
source code last updated 5/26/11
To Do: (Complete In Progress Not Started)
IN NO PERTICULAR ORDER...
- Create Enemies
- Bullet-Enemy/Bullet-Ship Interaction
- Level Progression
- GameOver
- Health PowerUp
- Money PowerUps
- Speed Modifier for School vs Home Testing
- Graphic Icons for New Weapons
- Temporary Fire Rate Increase PowerUp
- Temporary Invincibility PowerUp
- New Weapons
Sideways Shooting Mini-Bullets
Double-Bullets
Double-Bullets+Side-Bullets
Rapid-Fire Bullets (all bullets now fire faster)
Other...
- Weapon GUI
- Fix Weapon GUI Issues
- Raise/Lower Game Speed From Applet
- "Secret Somethings"
- Fix Fire Rate Issues
- Instructions
- Mute Button
- Weapon Store Between Levels
- Make Items Actually Purchasable
- Weapon switching while playing
- Better Variation Between Levels (meh.. it's good enough for now...)
- Convert to Applet
- Add Sound Effects
- More Sounds (collision sounds)
- Restructure current code into a more proessional format(thanks to Fr. Chris)
InvaderApplet Class
import java.awt.*; import java.applet.*; import java.awt.event.*; import java.awt.image.BufferedImage; import java.util.ArrayList; import javax.swing.*; import java.io.*; import javax.imageio.ImageIO; @SuppressWarnings("serial") public class InvaderApplet extends Applet implements ActionListener, KeyListener{ private Ship ship; //player controlled private Image img; //background image private Timer time; private Image healthImg; //heart image in bottom-left corner private Image single; private Image single2; private Image side; private Image side2; private Image doublee; private Image doublee2; private Image singleSide; private Image singleSide2; private Image doubleSide; private Image doubleSide2; private Image shipImage; private Image bow; private Image gameOverImage; private BufferedImage enemyImage; private BufferedImage bulletDownImage,bulletUpImage; private BufferedImage dropHealthImage,drop50Image, drop100Image,dropShieldImage,dropSpeedImage; private BufferedImage[] dropImgs; public final static int SINGLE=1;//normal weapon public final static int SIDE=3;//diagonal shooting public final static int DOUBLE=2;//double star shooting public final static int SINGLE_SIDE=4;//side stars+normal star public final static int DOUBLE_SIDE=5;//side stars+double center private int score=0; private int weapon = 1; private int money=0; //used to buy weapons private int currentUpgrade =1; private int SPEED=2; //use faster() and slower() to change private int delay =5000/SPEED; //time from starting program to start of game private int delayBeforeStore; //time between killing last enemy and shop/new-level screen private int theTime=0; //timer increases this variable private int shield; private int speedy; private int hits=0; private int misses=0; private int accuracy; private int level=1; //determines difficulty of enemies private boolean gameOver = false; //once health and lives are both depleted private int fireSpeed = 0; //limits how fast you can fire private int dmgDelay = 0; //prevents a single bullet from hitting a ship numerous times private ArrayList<Integer> keys = new ArrayList<Integer>(); //contains a list of keys being pressed private ArrayList<Bullet> bullets = new ArrayList<Bullet>(); //contains all bullets being shown private ArrayList<Enemy> enemies = new ArrayList<Enemy>(); //contains all enemies being shown private ArrayList<EnemyDrop> drops = new ArrayList<EnemyDrop>(); //contains all drops from killed enemies Image virtualMem; Graphics gBuffer; private AudioClip shoot; private AudioClip music; private AudioClip ting; private AudioClip chaChing; private AudioClip hit1; private AudioClip hit2; private boolean unMuted = true; private boolean cheater =false; public void init() { int width=getWidth(); int height=getHeight(); loadImageFiles(); ship = new Ship(width,height, shipImage); dropImgs = new BufferedImage[5]; dropImgs[0]=dropHealthImage; dropImgs[1]=drop50Image; dropImgs[2]=drop100Image; dropImgs[3]=dropSpeedImage; dropImgs[4]=dropShieldImage; virtualMem = createImage(width,height); gBuffer = virtualMem.getGraphics(); shoot = getAudioClip(getClass().getResource("/Shoot.wav")); music = getAudioClip(getClass().getResource("/GameSong.wav")); ting = getAudioClip(getClass().getResource("/ting.wav")); chaChing = getAudioClip(getClass().getResource("/chaChing.wav")); hit1 = getAudioClip(getClass().getResource("/hit1.wav")); hit2 = getAudioClip(getClass().getResource("/hit2.wav")); for(int i =0;i<7;i++) { enemies.add(new Enemy(20+i*70,45, enemyImage)); //adds first wave of enemies } addKeyListener(this); setFocusable(true); //music.loop(); time = new Timer(15, this); //creating the timer time.addActionListener(this); time.start(); //starting the timer } public void loadImageFiles(){ try { img = ImageIO.read(getClass().getResource("/starry-night-twinkle-sparkle.gif")); healthImg = ImageIO.read(getClass().getResource("/Health.gif")); single =ImageIO.read(getClass().getResource("/Normal_Bullet.gif")); side = ImageIO.read(getClass().getResource("/OnlySide.gif")); doublee = ImageIO.read(getClass().getResource("/DoubleStar.gif")); singleSide = ImageIO.read(getClass().getResource("/SideBullets.gif")); doubleSide = ImageIO.read(getClass().getResource("/DoubleStar_Side.gif")); single2 = ImageIO.read(getClass().getResource("/Normal_Bullet_Selected.gif")); side2 = ImageIO.read(getClass().getResource("/sidesel.gif")); doublee2 = ImageIO.read(getClass().getResource("/DoubleStar_Selected.gif")); singleSide2 = ImageIO.read(getClass().getResource("/SideBullets_Selected.gif")); doubleSide2 = ImageIO.read(getClass().getResource("/DoubleStar_Side_Selected.gif")); shipImage = ImageIO.read(getClass().getResource("/space_invaders_my_ship.gif")); enemyImage = ImageIO.read(getClass().getResource("/Enemy_Ship.gif")); bulletDownImage = ImageIO.read(getClass().getResource("/Silk_bullet_star.png")); bulletUpImage = ImageIO.read(getClass().getResource("/Silk_bullet_star_silver.png")); bow = ImageIO.read(getClass().getResource("/bow.gif")); dropHealthImage = ImageIO.read(getClass().getResource("/1Health.gif")); drop50Image = ImageIO.read(getClass().getResource("/50.gif")); drop100Image = ImageIO.read(getClass().getResource("/100.gif")); dropShieldImage = ImageIO.read(getClass().getResource("/shield.gif")); dropSpeedImage = ImageIO.read(getClass().getResource("/speedy.gif")); gameOverImage = ImageIO.read(getClass().getResource("/images.gif")); } catch (IOException e) { e.printStackTrace(); } } public void actionPerformed(ActionEvent e) { if(misses!=0){ accuracy=((hits/(hits+misses))*100); } if(keys.contains(KeyEvent.VK_A)||keys.contains(KeyEvent.VK_LEFT)) ship.moveLeft(SPEED); //tells ship to move left when a or left is held if(keys.contains(KeyEvent.VK_D)||keys.contains(KeyEvent.VK_RIGHT)) ship.moveRight(SPEED); //tells ship to move right when d or right is held if(keys.contains(KeyEvent.VK_W)||keys.contains(KeyEvent.VK_UP)) ship.moveUp(SPEED); //tells ship to move up when w or up is held if(keys.contains(KeyEvent.VK_S)||keys.contains(KeyEvent.VK_DOWN)) ship.moveDown(SPEED); //tells ship to move down when s or down is held if(keys.contains(KeyEvent.VK_1)) weapon=1; //switches to weapon 1 when 1 is pressed if(keys.contains(KeyEvent.VK_2)&¤tUpgrade>=2) weapon=2; //switches to weapon 2 when 2 is pressed if(keys.contains(KeyEvent.VK_3)&¤tUpgrade>=3) weapon=3; //switches to weapon 3 when 3 is pressed if(keys.contains(KeyEvent.VK_4)&¤tUpgrade>=4) weapon=4; //switches to weapon 4 when 4 is pressed if(keys.contains(KeyEvent.VK_5)&¤tUpgrade>=5) weapon=5; //switches to weapon 5 when 5 is pressed if(keys.contains(KeyEvent.VK_6)&¤tUpgrade>=6) weapon=6; //switches to weapon 6 when 6 is pressed if(keys.contains(KeyEvent.VK_C)&&keys.contains(KeyEvent.VK_B)&&keys.contains(KeyEvent.VK_A)){ money=9999999; currentUpgrade=6; cheater=true; } if(keys.contains(KeyEvent.VK_SPACE) && fireSpeed<1 && delay <=0){ if(weapon==SINGLE){ //creates weapon 1's bullets bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7,ship.getY(),Bullet.UP, bulletUpImage)); fireSpeed = 50; if(unMuted)shoot.play(); } if(weapon==DOUBLE){ //creates weapon 2's bullets bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7-10,ship.getY(),Bullet.UP, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7+10,ship.getY(),Bullet.UP, bulletUpImage)); fireSpeed = 60; if(unMuted)shoot.play(); } if(weapon==SIDE){//creates weapon 3's bullets bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7,ship.getY(),Bullet.UP,Bullet.LEFTY, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7,ship.getY(),Bullet.UP,Bullet.RIGHTY, bulletUpImage)); fireSpeed = 65; if(unMuted)shoot.play(); } if(weapon==SINGLE_SIDE){//creates weapon 4's bullets bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7,ship.getY(),Bullet.UP, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7,ship.getY(),Bullet.UP,Bullet.LEFTY, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7,ship.getY(),Bullet.UP,Bullet.RIGHTY, bulletUpImage)); fireSpeed =65; if(unMuted)shoot.play(); } if(weapon==DOUBLE_SIDE){//creates weapon 5's bullets bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7,ship.getY(),Bullet.UP,Bullet.LEFTY, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7,ship.getY(),Bullet.UP,Bullet.RIGHTY, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7-10,ship.getY(),Bullet.UP, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7+10,ship.getY(),Bullet.UP, bulletUpImage)); fireSpeed =75; if(unMuted)shoot.play(); } if(weapon==6){ bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7,ship.getY(),Bullet.UP,Bullet.LEFTY, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7,ship.getY(),Bullet.UP,Bullet.RIGHTY, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7+4,ship.getY()+2,Bullet.UP,Bullet.LEFTY, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7-4,ship.getY()+2,Bullet.UP,Bullet.RIGHTY, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7-2,ship.getY()+4,Bullet.UP,Bullet.LEFTY, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7+2,ship.getY()+4,Bullet.UP,Bullet.RIGHTY, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7-10,ship.getY()+2,Bullet.UP, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7+10,ship.getY()+2,Bullet.UP, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7-5,ship.getY()-2,Bullet.UP, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7+5,ship.getY()-2,Bullet.UP, bulletUpImage)); bullets.add(new Bullet((ship.getX()+ship.getWidth()/2)-7,ship.getY()-4,Bullet.UP, bulletUpImage)); fireSpeed =20; if(unMuted)shoot.play(); } } if(delay<=0){ reviewEnemies(); newCheck(); reviewBullets(); reviewDrops(); if(ship.getHealth()<=0){ if(ship.getLives()>0)ship.setHealth(3); ship.setLives(ship.getLives()-1); } } if(delay==0)music.loop(); if(!gameOver)repaint(); //calls paint delay--; } public void paint(Graphics g) { addCount(); //super.paint(g); if(speedy>0)fireSpeed-=2*SPEED; else{fireSpeed-=SPEED;} dmgDelay-=SPEED; delayBeforeStore-=SPEED; shield-=SPEED; speedy-=SPEED; Graphics2D g2d = (Graphics2D) gBuffer; g2d.drawImage(img, 0, 0, null); if(delay<=0){ if(speedy>=1){ g2d.setColor(Color.GREEN); g2d.drawString("Rapid-fire for: "+((speedy/100)+1), 190, 250); g2d.setColor(Color.WHITE); } if(shield>=1){ g2d.setColor(Color.CYAN); g2d.drawString("Shielded for: "+((shield/100)+1), 190, 262); g2d.setColor(Color.WHITE); } for(Enemy e: enemies) g2d.drawImage(e.getImage(),e.getX(),e.getY(),null); for(Bullet b:bullets) g2d.drawImage(b.getImage(),b.getX(),b.getY(),null); for(EnemyDrop ed:drops) ed.draw(g2d); g2d.drawImage(single2,20,10,null); if(currentUpgrade>=2)g2d.drawImage(side2,60,10,null); if(currentUpgrade>=3)g2d.drawImage(doublee2,100,10,null); if(currentUpgrade>=4)g2d.drawImage(singleSide2,140,10,null); if(currentUpgrade>=5)g2d.drawImage(doubleSide2,180,10,null); if(weapon==1)g2d.drawImage(single,20,10,null); else if(weapon==2)g2d.drawImage(side,60,10,null); else if(weapon==3)g2d.drawImage(doublee,100,10,null); else if(weapon==4)g2d.drawImage(singleSide,140,10,null); else if(weapon==5)g2d.drawImage(doubleSide,180,10,null); else if(weapon==6)g2d.drawImage(bow,220,10,null); g2d.setColor(Color.WHITE); //g2d.drawString("Weapon:"+weapon, 20,10); g2d.drawString("Health:", 20, 460); if(ship.getHealth()==5)g2d.drawImage(healthImg,20,465,null); else if(ship.getHealth()==4)g2d.drawImage(healthImg,20,465,20+47,465+13,0,0,47,13,null); else if(ship.getHealth()==3)g2d.drawImage(healthImg,20,465,20+36,465+13,0,0,36,13,null); else if(ship.getHealth()==2)g2d.drawImage(healthImg,20,465,20+24,465+13,0,0,24,13,null); else if(ship.getHealth()==1)g2d.drawImage(healthImg, 20, 465,20+12,465+13,0,0,12,13,null); g2d.drawImage(ship.getImage(), ship.getX(), ship.getY(), null); g2d.drawString("Lives:",275,472); g2d.drawString("Score: "+score, 150, 460); g2d.drawString("Money: "+money, 150, 475); for(int i = ship.getLives();i>0;i--){ g2d.drawImage(ship.getImage(),475-(30*i),450,null); } if(ship.getLives()<=0 && ship.getHealth()<=0){ //GAMEOVER gameOver=true; music.stop(); g2d.drawImage(gameOverImage,0,0,512,512,null); g2d.drawString("Your score was: "+score,185,250); //g2d.drawString("Your accuracy was: "+accuracy+"%",185,265); } } else{ //SHOP g2d.setColor(Color.WHITE); g2d.drawString("Level "+level+" will begin in:",190,235); g2d.drawString(Integer.toString(delay/100),250,256); g2d.drawString("(press ENTER to start now)", 172, 277); g2d.drawString("By: Cameron Aubert", 350, 500); int x =400; g2d.drawString("Controls:",60,x-15); g2d.drawString("Move Up: W / UP",15,x); g2d.drawString("Move Down: S / DOWN",15,15+x); g2d.drawString("Move Left: A / LEFT",15,30+x); g2d.drawString("Move Right: D / RIGHT",15,45+x); g2d.drawString("Shoot: SPACE",15,60+x); g2d.drawString("Weapons: 1/2/3/4/5",15,75+x); g2d.drawString("Toggle Sound: M",15,90+x); g2d.drawString("Current Cash: "+money,350,30); if(cheater)g2d.drawString("Cheater!",350, 15); if(currentUpgrade==1 && money>=1500)g2d.setColor(Color.WHITE); else{ g2d.setColor(Color.DARK_GRAY); } g2d.drawString("(U) Upgrade to Double Bullets for $1500",15,20); if(currentUpgrade==2 && money>=1000)g2d.setColor(Color.WHITE); else{ g2d.setColor(Color.DARK_GRAY); } g2d.drawString("(U) Upgrade to Side Bullets for $1000",15,35); if(currentUpgrade==3 && money>=1500)g2d.setColor(Color.WHITE); else{ g2d.setColor(Color.DARK_GRAY); } g2d.drawString("(U) Upgrade to Normal + Side Bullets for $1500",15,50); if(currentUpgrade==4 && money>=2000)g2d.setColor(Color.WHITE); else{ g2d.setColor(Color.DARK_GRAY); } g2d.drawString("(U) Upgrade to Double + Side Bullets for $2000",15,65); if(ship.getHealth()<5 && money>=500)g2d.setColor(Color.WHITE); else{ g2d.setColor(Color.DARK_GRAY); } g2d.drawString("(H) Purchase Health ("+ship.getHealth()+"/5) for $500",15,80); if(ship.getLives()<5 && money>=2000)g2d.setColor(Color.WHITE); else{ g2d.setColor(Color.DARK_GRAY); } g2d.drawString("(L) Purchase Another Life ("+ship.getLives()+"/5) for $2000",15,95); //g2d.drawString("",15,110); } g.drawImage(virtualMem,0,0,this); } public void reviewEnemies(){ int i=0; while(i<enemies.size()){ Enemy e=enemies.get(i); for(Bullet b:bullets){ if(b.getDirection()==Bullet.UP && b.getRect().intersects(e.getRect())){ e.hit(); b.hit(); hit2.play(); hits++; } } if(theTime%(15/SPEED)==0)e.move(getWidth()); if(Math.random()<.004+(.0025*level) /*&& bullets.size()<200*/) bullets.add(new Bullet((e.getX()+e.getWidth()/2)-7,e.getY()+20,Bullet.DOWN, bulletDownImage)); if(Math.random()<.0001)drops.add(new EnemyDrop((e.getX()+e.getWidth()/2)-7,e.getY()+20,(int)((Math.random()*2)+4),dropImgs)); if(e.getHealth()<=0){ drops.add(new EnemyDrop((e.getX()+e.getWidth()/2)-7,e.getY()+20,dropImgs)); enemies.remove(e); score+=((level*100)/2); } else{ i++; } } } public void reviewBullets(){ int i=0; while(i < bullets.size()){ Bullet b=bullets.get(i); b.move(SPEED); if(b.getRect().intersects(ship.getRect())&&b.getDirection()==Bullet.DOWN){ b.hit(); if(dmgDelay<=0&&shield<=0){ ship.hit(); hit1.play(); dmgDelay=10; } } if(b.getY()<-20||b.getY()>525){ misses++; b.hit(); } if(b.hasHit()){ bullets.remove(b); }else{ i++; } } } public void reviewDrops(){ int i=0; while(i<drops.size()){ EnemyDrop ed=drops.get(i); if(theTime%2==0)ed.move(SPEED); if(ed.getRect().intersects(ship.getRect())){ ed.hit(); if(ed.getType()==1&&ship.getHealth()<5){ ship.setHealth(ship.getHealth()+1); if(unMuted)ting.play(); } else if(ed.getType()==1){ score+=500; if(unMuted)ting.play(); } if(ed.getType()==2){ money+=50; if(unMuted)chaChing.play(); } if(ed.getType()==3){ money+=100; if(unMuted)chaChing.play(); } if(ed.getType()==4){ if(speedy<1)speedy=1000; else{speedy+=1000;} if(unMuted)ting.play(); } if(ed.getType()==5){ if(shield<1)shield=1000; else{shield+=1000;} if(unMuted)ting.play(); } } if(ed.isHit()||ed.getY()>525){ drops.remove(ed); } else { i++; } } } public void newCheck(){ if(enemies.isEmpty()){ if(delayBeforeStore<-1000)delayBeforeStore=300/SPEED; if(delayBeforeStore<=0){ level++; for(int i =0;i<7;i++){ enemies.add(new Enemy(2+level,20+i*70,30, enemyImage)); } bullets.clear(); delay=2500/SPEED; music.stop(); } } } public void addCount(){ if(theTime >= Integer.MAX_VALUE-100) theTime=0; theTime++; } public void delay(int delay){ this.delay=delay; } public void keyTyped(KeyEvent arg0) { } public void keyPressed(KeyEvent e){ if(!keys.contains(e.getKeyCode())) keys.add(e.getKeyCode()); if(e.getKeyCode()==KeyEvent.VK_M){ unMuted=!unMuted; if (unMuted)music.loop(); if(!unMuted)music.stop(); } if(e.getKeyCode()==KeyEvent.VK_ENTER){ delay=0; } if(e.getKeyCode()==KeyEvent.VK_MINUS){ slower(); } if(e.getKeyCode()==KeyEvent.VK_EQUALS){ faster(); } if(e.getKeyCode()==KeyEvent.VK_U){ if(currentUpgrade==1&&money>=1500){ money-=1500; currentUpgrade++; weapon=2; } if(currentUpgrade==2&&money>=1000){ money-=1000; currentUpgrade++; weapon=3; } if(currentUpgrade==3&&money>=1500){ money-=1500; currentUpgrade++; weapon=4; } if(currentUpgrade==4&&money>=2000){ money-=2000; currentUpgrade++; weapon=5; } } if(e.getKeyCode()==KeyEvent.VK_H){ if(ship.getHealth()<5&&money>=500){ money-=500; ship.setHealth(ship.getHealth()+1); } } if(e.getKeyCode()==KeyEvent.VK_L){ if(ship.getLives()<5&&money>=2000){ money-=2000; ship.setLives(ship.getLives()+1); } } } public void faster(){ SPEED+=1; } public void slower(){ if(SPEED>1)SPEED-=1; } public void keyReleased(KeyEvent e){ keys.remove(new Integer(e.getKeyCode())); } public void update(Graphics g){ paint(g); } }
Ship Class
import java.awt.*; public class Ship{ private int lives; private int health; private int x; private int y; private int width; private int height; private Rectangle rect; private Image image; private int screenWidth, screenHeight; public Ship(int width, int height, Image img){ this(5,width/2-(35/2),height-35-60,35,35, img); this.setBounds(width, height); } public Ship(int lives, int x, int y, int width, int height, Image img){ this.lives=lives; this.x=x; this.y=y; this.width=width; this.height=height; rect=new Rectangle(x+5,y+6,width-10,height-10); this.image=img; health = 3; } public void setBounds(int w, int h){ screenWidth=w; screenHeight=h; } public void moveLeft(int x){ this.x-=x; keepInBounds(); } public void hit(){ health--; } public void moveRight(int x){ this.x+=x; keepInBounds(); } public void moveUp(int x){ y-=x; keepInBounds(); } public void moveDown(int x){ y+=x; keepInBounds(); } public Rectangle getRect(){ return rect; } public Image getImage(){ return image; } public void setLives(int health) { this.lives = health; } public int getLives() { return lives; } public void setX(int x) { this.x = x; } public int getX() { return x; } public void setY(int y) { this.y = y; } public int getY() { return y; } public int getHeight(){ return height; } public void setHeight(int height){ this.height=height; } public int getWidth(){ return width; } public void setWidth(int width){ this.width=width; } public void keepInBounds(){ if(x<10)x=10; if(y<100)y=100; if(x>screenWidth-width-10)x=screenWidth-width-10; if(y>screenHeight-height-60)y=screenHeight-height-60; rect.setLocation(x,y); if(health>5)health=5; } public void setHealth(int health) { this.health = health; } public int getHealth() { return health; } }
EnemyDrop Class
import java.awt.Graphics; import java.awt.Rectangle; import java.awt.image.BufferedImage; public class EnemyDrop { private int type; private int x; private int y; private Rectangle rect; private Boolean hit; private int height; private int width; final int HEALTHUP = 1; final int MONEY50 = 2; final int MONEY100 = 3; private BufferedImage image; public EnemyDrop(int x, int y, BufferedImage[] imgs) { setX(x); setY(y); this.type=(int)(Math.random()*3)+1; BufferedImage img=imgs[type-1]; this.image=img; setWidth(img.getWidth()); setHeight(img.getHeight()); setRect(new Rectangle(x,y,width,height)); setHit(false); } public void draw(Graphics g){ g.drawImage(image,x,y,null); } public void setType(int type) { this.type = type; } public int getType() { return type; } public void move(int speed){ y+=speed; rect.setLocation(x,y); } public void setX(int x) { this.x = x; } public int getX() { return x; } public void setY(int y) { this.y = y; } public int getY() { return y; } public void setRect(Rectangle rect) { this.rect = rect; } public Rectangle getRect() { return rect; } public void setHit(Boolean hit) { this.hit = hit; } public Boolean isHit() { return hit; } public void hit(){ hit=true; } public void setHeight(int height) { this.height = height; } public int getHeight() { return height; } public void setWidth(int width) { this.width = width; } public int getWidth() { return width; } }
Enemy Class
import java.awt.*; import java.awt.image.BufferedImage; public class Enemy { private BufferedImage image; private int x; private int y; private int health; private int width; private int height; private Rectangle rect; public Enemy(int x, int y, BufferedImage img){ this(2,x,y, img); } public Enemy(int health, int x, int y, BufferedImage img){ this.setHealth(health); this.setX(x); this.setY(y); image= img; height= image.getHeight(); width=image.getWidth(); setRect(new Rectangle(x+1,y+2,width-2,height-4)); } public void setImage(BufferedImage image) { this.image = image; } public void move(int screenWidth){ int random = (int) (Math.random()*4); if(random<1) x+=2; else if(random>=1 && random<2) x-=2; else if(random>=2 && random<3) y+=2; else{ y-=2; } keepInBounds(screenWidth); } public void keepInBounds(int screenWidth){ if(x<10)x=10; if(y<25)y=25; if(x>screenWidth-width-10)x=screenWidth-width-10; if(y>height+65)y=height+65; rect.setLocation(x,y); } public Image getImage() { return image; } public void setX(int x) { this.x = x; } public int getX() { return x; } public void setHealth(int health) { this.health = health; } public int getHealth() { return health; } public void setY(int y) { this.y = y; } public int getY() { return y; } public void setWidth(int width) { this.width = width; } public int getWidth() { return width; } public void setHeight(int height) { this.height = height; } public int getHeight() { return height; } public void moveLeft(int x){ this.x-=x; } public void moveRight(int x){ this.x+=x; } public void moveUp(int x){ y-=x; } public void moveDown(int x){ y+=x; } public void setRect(Rectangle rect) { this.rect = rect; } public void hit() { health--; } public Rectangle getRect() { return rect; } }
Bullet Class
import java.awt.*; import java.awt.image.BufferedImage; public class Bullet{ private int x; private int y; final static Boolean DOWN = false; final static Boolean UP = true; final static int LEFTY = 1; final static int RIGHTY = 2; private boolean direction; private Rectangle rect; protected int width; protected int height; protected BufferedImage image; private boolean hit; private int type; public Bullet(int x, int y, boolean direction, BufferedImage img){ setX(x); setY(y); setDirection(direction); setRect(new Rectangle(x+5,y+5,6,6)); image=img; width=img.getWidth(); height=img.getHeight(); setHit(false); } public Bullet(int x, int y, Boolean direction,int type, BufferedImage img){ setX(x); setY(y); setDirection(direction); image=img; width=img.getWidth(); height=img.getHeight(); setRect(new Rectangle(x,y,width,height)); setHit(false); this.type=type; } public void setHit(boolean b) { hit=b; } public void move(int speed){ if(type==LEFTY){ y-=2*speed; x-=speed; } else if(type == RIGHTY){ y-=2*speed; x+=speed; } else if(!direction)y+=speed; else if(direction)y-=2*speed; rect.setLocation(x,y); } public Image getImage(){ return image; } public int getHeight(){ return height; } public int getWidth(){ return width; } public void setX(int x) { this.x = x; } public int getX() { return x; } public void setY(int y) { this.y = y; } public int getY() { return y; } public void setRect(Rectangle rect) { this.rect = rect; } public Rectangle getRect() { return rect; } public void setDirection(boolean direction2) { this.direction = direction2; } public Boolean getDirection() { return direction; } public void hit() { hit=true; } public boolean hasHit() { return hit; } }
Files





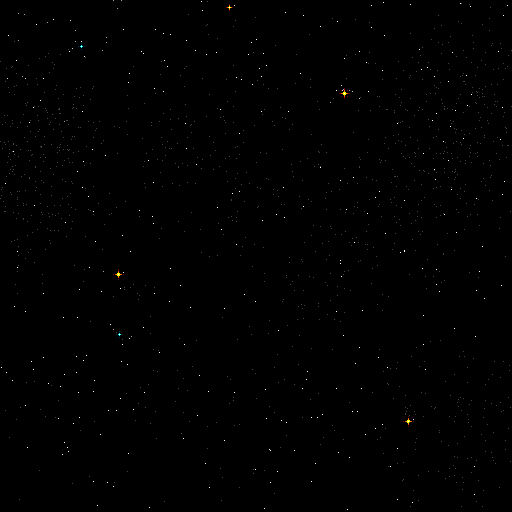










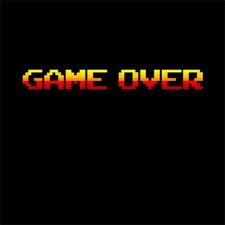


