Black Hole Critter
<< PigeonCritter | GridworldTrailIndex | Capture The Flag >>
See a Quicktime video of it in action
Make a Critter that forces all Actors in the grid to move towards it, and consumes any Actor that is adjacent to it. If you set the color of the BlackHole critter to null, it will show the colors of the gif file.
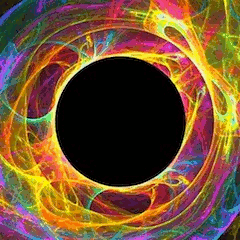
Since BlackHoleCritter is a subclass of Critter, you may not touch the act method. You adapt a Critter by changing one or more of the 5 methods that gets called by the act() method.
- public ArrayList<Actor> getActors()
- public void processActors(ArrayList<Actor> actors)
- public ArrayList<Location> getMoveLocations()
- public Location selectMoveLocation(ArrayList<Location> locs)
- public void makeMove(Location loc)
Use appendix C to see the specific Code and mind the pre- and post- conditions! First, find a way so it won't move. Next, find a way so all the Actors on the grid get selected so they can be processed. Finally, process the actors so that they move closer or are told "removeSelfFromGrid"
BlackHoleCritter.java
import info.gridworld.actor.*; import info.gridworld.grid.*; import java.util.ArrayList; public class BlackHoleCritter extends Critter { public BlackHoleCritter(){ super(); setColor(null); } }
BlackHoleCritterRunner.java
import info.gridworld.actor.*; import info.gridworld.grid.*; public class BlackHoleRunner { public static void main(String[] args) { ActorWorld world=new ActorWorld(); world.setGrid(new BoundedGrid<Actor>(20,20)); world.add(new Location(9,9), new BlackHoleCritter()); for (int i=0; i<50; i++){ world.add(new Critter()); world.add(new Rock()); } world.show(); } }
Things to Try
- specify a event horizon so if an Actor is a certain distance it is safe
- have it consume Actors that are within 2 Locations if its location.