Burgers
<< Field | OtherProjectsTrailIndex | Mr Potato Head >>
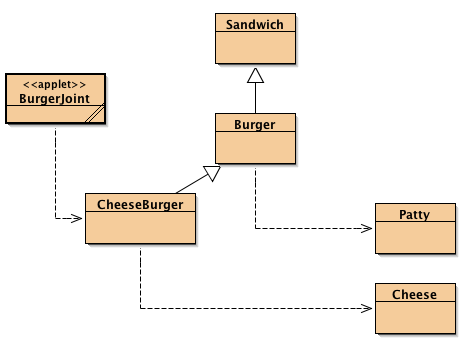
BurgerJoint.java
import java.awt.*; import java.applet.*; import javax.swing.JOptionPane; public class BurgerJoint extends Applet { int patties=1; public void init() { String orderUp = JOptionPane.showInputDialog("How many patties? "); patties=Integer.parseInt(orderUp); } public void paint(Graphics g) { CheeseBurger c=new CheeseBurger(g, patties ); } }
Patty.java
import java.awt.Graphics; class Patty { Color brown=new Color(100, 50, 0); public Patty(Graphics g, int location) { g.setColor(brown); g.fillRect(50, location, 300, 30); g.setColor(Color.orange); g.drawRect(50, location, 300, 30); } }
Cheese.java
import java.awt.Graphics; class Cheese { public Cheese(Graphics g, int location) { g.setColor(Color.yellow); Polygon cheese = new Polygon(); cheese.addPoint(50, location); cheese.addPoint(350, location); for (int i=350; i>55; i=i-(int)(30*Math.random())-10) cheese.addPoint(i, location+(int)(30*Math.random())); g.fillPolygon(cheese); } }
Sandwich.java
import java.awt.Graphics; class Sandwich { protected int layers, top; public Sandwich (Graphics g, int layers) { top=270-30*layers; g.setColor(Color.orange); //top bun g.fillArc(50, top-40, 300, 80, 0, 180); //bottom bun g.fillRect(50, 270, 300, 30); drawSeeds(g, top); } private void drawSeeds(Graphics g, int top) { g.setColor(Color.yellow); for(int i=0;i<30;i++) g.fillOval((int)(240*Math.random())+80, top-10-(int)(15*Math.random()), 3, 5); } public void drawLettice(Graphics g, int top) { g.setColor(Color.green); Polygon lettice = new Polygon(); lettice.addPoint(50, top); lettice.addPoint(350, top); for (int i=350; i>55; i=i-(int)(10*Math.random())) lettice.addPoint(i, top+(int)(30*Math.random())); g.fillPolygon(lettice); } }
Burger.java
import java.awt.Graphics; class Burger extends Sandwich { private Patty beef; public Burger (Graphics g, int layers) { super(g, layers); for (int p=0; p<layers; p++) beef=new Patty(g, top+30*p); drawLettice(g, top); } }
CheeseBurger.java
import java.awt.Graphics; class CheeseBurger extends Burger { private Cheese c; public CheeseBurger (Graphics g, int layers) { super(g, layers); for (int i=0;i<layers;i++) c=new Cheese(g, 240-i*30); drawLettice(g,top); } }