Mr Potato Head
<< Burgers | OtherProjectsTrailIndex | Morse Code Tone >>
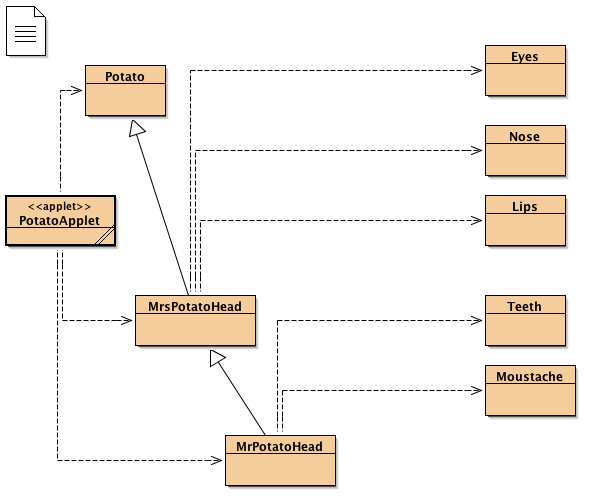
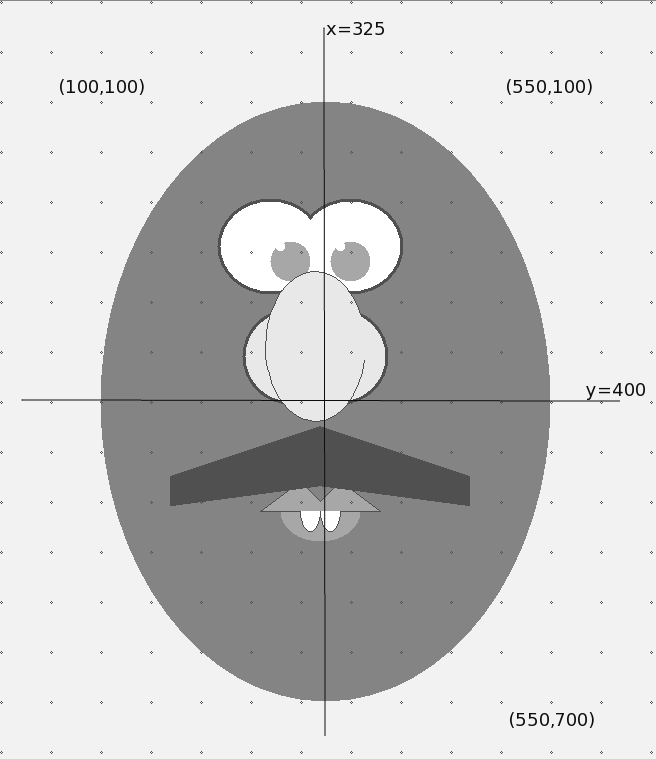
PotatoApplet.java
import java.awt.*; import java.applet.*; public class PotatoApplet extends Applet { public void paint(Graphics g) { Potato p = new MrPotatoHead(g); drawGrid(g); } public void drawGrid(Graphics g) { g.setColor(Color.BLACK); for(int i=0; i<getWidth(); i+=50) for(int j=0; j<getHeight(); j+=50) g.drawOval(i,j,2,2); } }
Potato.java
import java.awt.*; class Potato { Color brown=new Color(100,50,50); public Potato(Graphics g) { g.setColor(brown); g.fillOval(100,100,450,600); } }
MrsPotatoHead.java
import java.awt.*; class MrsPotatoHead extends Potato { private Eyes myEyes; private Nose myNose; private Lips myLips; public MrsPotatoHead(Graphics g) { super(g); myEyes = new Eyes(g,Color.BLUE, 220,200); myLips = new Lips(g, 320, 500); myNose = new Nose(g, 245,310); } }
MrPotatoHead.java
import java.awt.*; class MrPotatoHead extends MrsPotatoHead { private Teeth myTeeth; private Moustache myMoustache; public MrPotatoHead(Graphics g) { super(g); myMoustache = new Moustache(g, 320,425); myTeeth = new Teeth(g, 320, 500); } }
Eyes.java
import java.awt.*; public class Eyes { private int x,y; private Color c; /** * Constructor for objects of class Eyes */ public Eyes(Graphics g,Color c, int x, int y) { this.x = x; this.y = y; this.c = c; draw(g); } public void draw(Graphics g) { g.setColor(Color.black); g.fillOval(x-3,y-3,106,96); g.fillOval(x+80-3,y-3,106,96); g.setColor(Color.white); g.fillOval(x,y,100,90); g.fillOval(x+80,y,100,90); g.setColor(c); g.fillOval(x+50,y+40,40,40); g.fillOval(x+110,y+40,40,40); g.setColor(Color.white); g.fillOval(x+55,y+40,10,10); g.fillOval(x+115,y+40,10,10); } }
Nose.java
import java.awt.*; public class Nose { private int x,y; private Color c; /** * Constructor for objects of class Eyes */ public Nose(Graphics g,Color c, int x, int y) { this.x = x; this.y = y; this.c = c; draw(g); } public Nose(Graphics g, int x, int y) { this(g, new Color(255,190,190), x,y); } public void draw(Graphics g) { g.setColor(Color.black); g.fillOval(x-3,y-3,96,96); g.fillOval(x+50-3,y-3,96,96); g.setColor(c); g.fillOval(x,y,90,90); g.fillOval(x+50,y,90,90); g.fillOval(x+20,y-40,100,150); g.setColor(Color.black); g.drawArc(x+20,y-40,100,150, -10, -325); } }
Lips.java
import java.awt.*; public class Lips { private int x,y; private Color c; Color pink= new Color(255,190,190); /** * Constructor for objects of class Eyes */ public Lips(Graphics g,Color c, int x, int y) { this.x = x; this.y = y; this.c = c; draw(g); } public Lips(Graphics g, int x, int y) { this.x = x; this.y = y; this.c = Color.RED; draw(g); } public void draw(Graphics g) { g.setColor(c); g.fillArc(x-40,y-20,80,60,0,-180); Polygon upperLip = new Polygon(); upperLip.addPoint(x,y); upperLip.addPoint(x-20,y-20); upperLip.addPoint(x-60,y+10); upperLip.addPoint(x,y+10); upperLip.addPoint(x+60,y+10); upperLip.addPoint(x+20,y-20); g.fillPolygon(upperLip); g.setColor(Color.black); g.drawPolygon(upperLip); } }
Teeth.java
import java.awt.*; public class Teeth { private int x,y; private Color c; Color pink= new Color(255,190,190); /** * Constructor for objects of class Eyes */ public Teeth(Graphics g,Color c, int x, int y) { this.x = x; this.y = y; this.c = c; draw(g); } public Teeth(Graphics g, int x, int y) { this.x = x; this.y = y; this.c = Color.WHITE; draw(g); } public void draw(Graphics g) { g.setColor(c); g.fillArc(x-20,y-10,20,40,0,-180); g.fillArc(x,y-10,20,40,0,-180); g.setColor(Color.black); g.drawArc(x-20,y-10,20,40,0,-180); g.drawArc(x,y-10,20,40,0,-180); } }
Moustache.java
import java.awt.*; public class Moustache { private int x,y; private Color c; Color pink= new Color(255,190,190); /** * Constructor for objects of class Eyes */ public Moustache(Graphics g,Color c, int x, int y) { this.x = x; this.y = y; this.c = c; draw(g); } public Moustache(Graphics g, int x, int y) { this.x = x; this.y = y; this.c = Color.BLACK; draw(g); } public void draw(Graphics g) { Polygon moustache = new Polygon(); moustache.addPoint(x,y); moustache.addPoint(x+150,y+50); moustache.addPoint(x+150,y+80); moustache.addPoint(x,y+60); moustache.addPoint(x-150,y+80); moustache.addPoint(x-150,y+50); g.setColor(c); g.fillPolygon(moustache); } }