Draw X
<< ImageViewer | SquintTrailIndex | Dimmer with image filters >>
Lets draw an X on an image. Each image has an 2-D array with a brightness from 0 to 255, where 0 is black and 255 is white. If the image is color, there are 3 arrays, one for Red, another for Green, the last for Blue. Combinations of red green and blue can compose practically any color. Lets start by getting the array, and switching certain pixels values to black (0) or white (255)
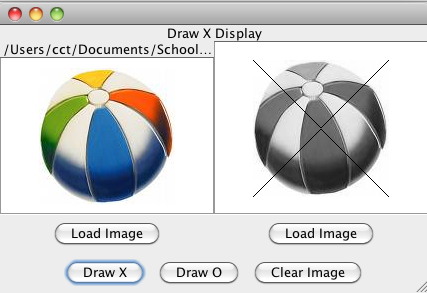
DrawXDisplay.java
import squint.*; // See http://www.cs.williams.edu/~tom/weavingCS/s07/doc/squintDoc/squint/GUIManager.html import javax.swing.*; import java.awt.*; /** * DrawXDisplay intorduces the idea of a 2D pixel array, as well as adding images and buttons. * * @author Chris Thiel * @version 16 Jan 2010 */ public class DrawXDisplay extends GUIManager { private ImageViewer originalImage = new ImageViewer(); private ImageViewer newImage = new ImageViewer(); private JButton drawXButton = new JButton("Draw X"); private JButton clearButton = new JButton("Clear Image"); /** * Dimmer DIsplay is the second Squint Lab */ public DrawXDisplay() { contentPane.setLayout( new BorderLayout() ); contentPane.add(new JLabel("Draw X Display", JLabel.CENTER), BorderLayout.NORTH); originalImage.setPic(new SImage(100,100,128)); JPanel imagePanel = new JPanel(new GridLayout(1,0)); imagePanel.add(originalImage); imagePanel.add(newImage); contentPane.add(imagePanel, BorderLayout.CENTER); JPanel buttonPanel = new JPanel(); buttonPanel.add(drawXButton); buttonPanel.add(clearButton); contentPane.add(buttonPanel, BorderLayout.SOUTH); } public void buttonClicked( JButton which ) { if(which == drawXButton){ newImage.setPic(drawX ( originalImage.getPic() )); } else if (which == clearButton){ originalImage.setPic(new SImage(100,100,128)); newImage.setPic(new SImage(100,100,255)); } } public SImage drawX( SImage oldPic ) { int [][] pixels = oldPic.getPixelArray(); int width=pixels.length; int height=pixels[0].length; for(int i=0; i<width; i++) pixels[i][i] = 0; // your code here return new SImage(pixels); } }
Squint2App.java
/** * SqunitApp2 has the main method * to start up the DrawXDisplay. * * @author Chris Thiel * @version 23 Jan 2010 */ public class Squint2App { public static void main(String[] args) { DrawXDisplay squint2=new DrawXDisplay(); squint2.createWindow(1200,600); } }
Activities
- Only one diagonal works. Figure out how to draw the other diagonal. Just use the default image for now.
- Make the X white rather than black
- This only works on square images. Find a way so it will work on Rectangular Images. This can be tricky, so here is a hint
- X is getting boring. Add a button to draw a different shape like a rectangular border 10 pixels from the edge
- Draw a Circle. recall {
⚠ $x=r \cos \theta$
} and {⚠ $y= r \sin \theta$
} You can offset the circle to the center by adding half the width to the x co-ordinate and half the height to the y co-ordinate. You may wish to have the radiusdouble r=(double)Math.min(height,width)/4.0;
