How To Put A Running Version Of Your Project On The Web
<< MoveImage | FinalProjectsTrailIndex | How to convert your Applet to an Application >>
Adapt your Code
If you use image files or textiles, make sure that they are in your project. In order that your applet can find them in the jar file, you have to specify the location of the class:
public Image getImage(String imageFileName) { URL imageURL = getClass().getResource(imageFileName); if (imageURL == null) { // handle the error, for example: // System.out.println( "Drat! "+imageFileName+" not found"); return null; }
or with a text file
public boolean readFile(String fileName, ArrayList<String> list){ try { InputStream in = getClass().getResourceAsStream(fileName); BufferedReader inStream =new BufferedReader(new InputStreamReader(in)); String nextLine; while( (nextLine = inStream.readLine() ) !=null) list.add(nextLine); inStream.close(); return true; } catch(IOException e) { list.add("Drat! An error has occurred \n"+e.getMessage()); return false; } }
Eclipse Tip
You can drag your sound and graphics files from the desktop into your project folder. If they are put their manually, you can make sure that they are registered and packed into your jar file by going to the project's "Properties" (Ctrl-I or Cmd-I), Select "Java Build Path" on the left, select the "Libraries" tab on the right, then press the "Add Class Folder" button to select the folder that has your resources. After pressing OK a few times, you'll notice the the folder will have a different mini-icon and a "Referenced Libraries" folder.
Make a jar file from your project
Place all your sound and image files in your project folder, and under BlueJ's "Project" Folder, select "Make a jar file". Name it The same as your applet's class. For example if Hockey.java is the class that extends Applet (or JApplet), Name the Jar file Hockey.jar
Write a HTML file (the web page)
like this one:
<html> <head> <title>Big Bird Applet</title> </head> <body> <h1>Big Bird Applet</h1> <h2>by Elmo</h2> <hr> <applet code="BigBird.class" width=800 height=600 archive="BigBird.jar" alt="Your browser understands the <APPLET> tag but isn't running the applet, for some reason." > Your browser is ignoring the <APPLET> tag! </applet> <hr> </body> </html>
and name it "BigBird.html" or "BigBIrd.htm"
Post the jar file and the Html file to your web server.
You will notice that there still may be problems since your code needs to access the the resource files (sounds and graphics) within the jar file. To help, make sure you have enabled the Java Cosole so you can read the details of any error messages,
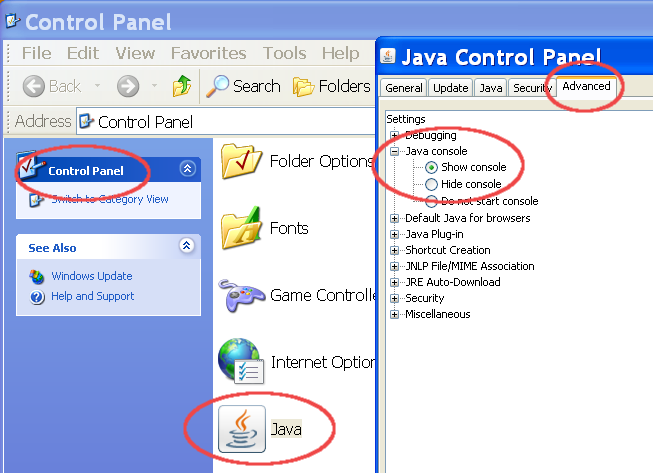
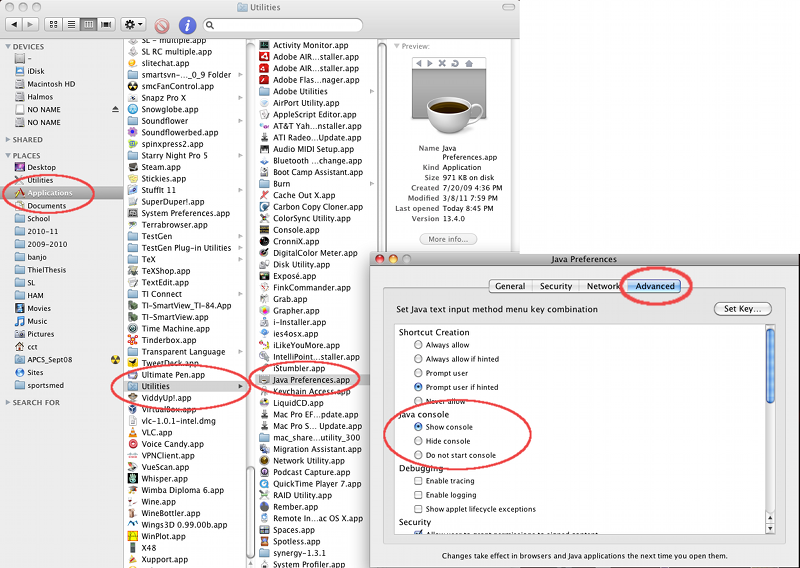