Move Image
<< BoxMover | FinalProjectsTrailIndex | How To Put A Running Version Of Your Project On The Web >>
Move an Image in your Applet
Link to the Application Version

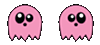
The monster class Makes an object that keeps track of its image and where it is on the screen. You need to same the image file (preferably a gif file with a transparent background) to the same folder that your project's '.class' files exist (either the project folder or the 'bin' folder of your project's folder).
The next three classes demonstrate how to move the monster either by Key presses, or by MouseMotion events that moves with the mouse (good for paddle movement) and another that moves the Monster with a click of the mouse (good for firing)
Monster.java
import java.awt.Graphics; import java.awt.image.BufferedImage; import java.io.File; import java.io.IOException; import javax.imageio.ImageIO; public class Monster { private int x; private int y; boolean left; private BufferedImage img; // see http://download.oracle.com/javase/tutorial/2d/images/loadimage.html public Monster(String graphicFileName, int x, int y){ this.x=x; this.y=y; left=false; this.img = null; try { img = ImageIO.read(new File(graphicFileName)); } catch (IOException e) { } } public int getX() {return x;} public int getY() {return y;} /** * if x changes, we might need to switch the direction our monster is facing * @param x */ public void setX(int x){ left=true; if (x>this.x){ left=false; } this.x=x; } public void setY(int y){ this.y=y;} // see http://download.oracle.com/javase/tutorial/2d/images/drawimage.html /** * this is a basic way of drawing the whole img, as it is */ public void draw(Graphics g){ g.drawImage(img, x, y, null); } /** * this uses a "doubled" graphic where the left image is on the left and the right image is on the right * @param g */ public void draw2(Graphics g){ if (left){ g.drawImage(img, x, y, x+img.getWidth()/2, y+img.getHeight(), 0, 0, img.getWidth()/2, img.getHeight(), null); }else { g.drawImage(img, x, y, x+img.getWidth()/2, y+img.getHeight(), img.getWidth()/2, 0, img.getWidth(), img.getHeight(), null); } } }
MoveImageWithKeys.java
import java.applet.Applet; import java.awt.Graphics; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; /** * Demonstrates drawing an image and * using the arrow keys to move up and down, left and right * @author Chris Thiel, OFMCap * size of applet should be 800 x 600 */ @SuppressWarnings("serial") public class MoveImageWithKeys extends Applet implements KeyListener { public static final int amount=5; //amount of change each arrow will change private Monster monster; public void init(){ monster = new Monster("pinky2.gif", 20, 100); //or for single image use: //monster = new Monster("pinky.gif", 20, 100); this.addKeyListener(this); } public void paint(Graphics g){ g.drawString("Click Screen for focus. Use Arrows to view left and right, up and down", 20, 20); monster.draw2(g); // or for single image use: // monster.draw(g); } @Override public void keyPressed(KeyEvent e) { int keyCode=e.getKeyCode(); if(keyCode==38){ //up monster.setY(monster.getY()-amount); } else if (keyCode==40){ //down monster.setY(monster.getY()+amount); } else if (keyCode==37){ //left monster.setX(monster.getX()-amount); } else if (keyCode==39){ //right monster.setX(monster.getX()+amount); }else if (keyCode==65){ //forward } repaint(); } @Override public void keyReleased(KeyEvent e) {} @Override public void keyTyped(KeyEvent e) {} }
MoveImageWithMouseMotion.java
import java.applet.Applet; import java.awt.Graphics; import java.awt.event.MouseEvent; import java.awt.event.MouseMotionListener; /** * Demonstrates drawing an image and * using the mouse to move the image * @author Chris Thiel, OFMCap * size of applet should be 800 x 600 */ @SuppressWarnings("serial") public class MoveImageWithMouse extends Applet implements MouseMotionListener { private Monster monster; public void init(){ monster = new Monster("pinky2.gif", 20, 100); //or for single image use: //monster = new Monster("pinky.gif", 20, 100); this.addMouseMotionListener(this); } public void paint(Graphics g){ g.drawString("Click Screen for focus. Monster follows your mouse", 20, 20); monster.draw2(g); // or for single image use: // monster.draw(g); } @Override public void mouseDragged(MouseEvent e) {} @Override public void mouseMoved(MouseEvent e) { monster.setX(e.getX()); monster.setY(e.getY()); repaint(); } }
MoveImageWithMouse.java
import java.applet.Applet; import java.awt.Graphics; import java.awt.event.MouseEvent; import java.awt.event.MouseListener; /** * Demonstrates drawing an image and * using the mouse to move the image * @author Chris Thiel, OFMCap * size of applet should be 800 x 600 */ @SuppressWarnings("serial") public class MoveImageWithMouse extends Applet implements MouseListener { private Monster monster; public void init(){ monster = new Monster("pinky2.gif", 20, 100); //or for single image use: //monster = new Monster("pinky.gif", 20, 100); this.addMouseListener(this); } public void paint(Graphics g){ g.drawString("Click Screen for focus. Click to move monster", 20, 20); monster.draw2(g); // or for single image use: // monster.draw(g); } @Override public void mouseClicked(MouseEvent e) {} @Override public void mouseEntered(MouseEvent e) {} @Override public void mouseExited(MouseEvent e) {} @Override public void mousePressed(MouseEvent e) {} @Override public void mouseReleased(MouseEvent e) { monster.setX(e.getX()); monster.setY(e.getY()); repaint(); } }