Image Movement Application
<< Keyboard Listener Application | Applications | Move a Monster Application >>
Download and place these image files in the same folder of your project.
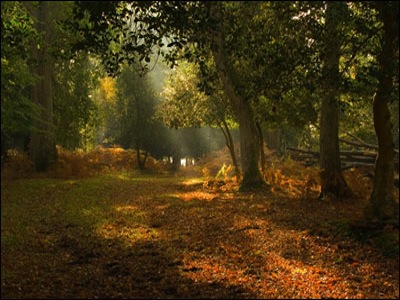

import java.awt.Color; import java.awt.Font; import java.awt.Graphics; import java.awt.Toolkit; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; import javax.swing.JFrame; import javax.swing.JPanel; import java.awt.image.*; import java.net.URL; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import java.io.IOException; import javax.imageio.ImageIO; public class MovePictureApplication extends JPanel implements KeyListener { public static int WIDTH=400; public static int HEIGHT=300; private int keyCode; private char c; private int x, y; private Font font; private BufferedImage background, butterfly; /** * imgBuffer is the "off screen" graphics area * gBuffer is imgBuffer's graphics object * which we need to draw stuff on the image */ private BufferedImage imgBuffer; private Graphics gBuffer; public MovePictureApplication() { //initialize variables here... keyCode=0; c='-'; background=loadImage("background.jpg"); butterfly=loadImage("butterfly.gif"); x = WIDTH/2; y = HEIGHT/2; font = new Font("Helvetica", Font.BOLD, 18); } public BufferedImage loadImage(String imageFileName) { URL url = getClass().getResource(imageFileName); if (url == null) throw new RuntimeException("cannot find file: " + imageFileName); try { return ImageIO.read(url); } catch(IOException e) { throw new RuntimeException("unable to read from file: " + imageFileName); } } public static void main(String[] args) { MovePictureApplication app= new MovePictureApplication(); JFrame window = new JFrame("Type a Key to See Its Code"); window.setSize(WIDTH, HEIGHT); window.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); window.getContentPane().add(app); window.addKeyListener(app); //window.pack(); window.setVisible(true); } public void paintComponent(Graphics g){ super.paintComponent(g); g.setColor(Color.WHITE); g.setFont(font); g.drawString("Type a Key", 20, 20); //g.setColor(Color.BLACK); //g.drawString("Version 1.0", 20, 40); //g.drawString("the key char "+c+" has code "+keyCode, 20, 60); //g.setFont(new Font("Arial", Font.PLAIN, 48)); //g.setColor(Color.red); //g.drawString("\""+c+"\"",80, 120); if (background!=null){ g.drawImage(background, 0, 0, WIDTH, HEIGHT, null); } else { g.setColor(Color.WHITE); g.fillRect(0, 0, WIDTH, HEIGHT); g.setColor(Color.RED); g.drawString("background image not found", 20, 60); } if (butterfly!=null){ g.drawImage(butterfly, x, y, null); } else { g.setColor(Color.CYAN); g.fillOval(x, y, 50, 50); g.setColor(Color.RED); g.drawString("butterfly image not found", 20, 80); } g.setColor(Color.CYAN); g.setFont(font); g.drawString("X = "+x, 20, 40); } // update is a workaround to cure Windows screen flicker problem public void update(Graphics g){ paint(g); } // These 3 methods need to be declares to implement the KeyListener Interface @Override public void keyTyped(KeyEvent e) {} @Override public void keyPressed(KeyEvent e) { keyCode=e.getKeyCode(); c=e.getKeyChar(); int dx = (int)(Math.random()*4)-2; int dy = (int)(Math.random()*4)-2; x+=dx; y+=dy; repaint(); } @Override public void keyReleased(KeyEvent e) {} }