Move A Monster Application
<< Image Movement Application | Applications | Mouse Listener Application >>

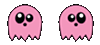
The Monster object can have a image or not. There are two different applications: one which moves the Monster, the other has a movable Monster that avoids falling monsters.
Monster.java
import java.awt.Color; import java.awt.Graphics; import java.awt.Rectangle; import java.awt.image.BufferedImage; import java.io.IOException; import java.net.URL; import javax.imageio.ImageIO; public class Monster { private BufferedImage image; private int x,y; public Monster() { x=20; y=20; image=null; } public Monster(String imageFileName) { this(); image = loadImage(imageFileName); } public Monster(int x, int y) { this.x=x; this.y=y; image=null; } public Monster(int x, int y, String imageFileName) { this(x,y); image = loadImage(imageFileName); } public void draw(Graphics g){ if (image==null){ g.setColor(Color.BLUE); g.fillOval(x, y, 10, 10); }else{ g.drawImage(image, x, y, null); } } public void up(int amt){ y-=amt; } public void down(int amt){ y+=amt; } public void left(int amt){ x-=amt; } public void right(int amt){ x+=amt; } public Rectangle getRectangle(){ int height=10; int width=10; if(image!=null){ height=image.getHeight(); width=image.getWidth(); } return new Rectangle(x,y,width, height); } public boolean collides(Rectangle box){ return box.intersects(getRectangle()); } public BufferedImage loadImage(String imageFileName) { URL url = getClass().getResource(imageFileName); if (url == null) throw new RuntimeException("cannot find file: " + imageFileName); try { return ImageIO.read(url); } catch(IOException e) { throw new RuntimeException("unable to read from file: " + imageFileName); } } public int getX(){return x;} public int getY(){return y;} public void setX(int x){this.x=x;} public void setY(int y){this.y=y;} }
MonsterMove.java
import java.awt.Color; import java.awt.Font; import java.awt.Graphics; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; import javax.swing.JFrame; import javax.swing.JPanel; public class MonsterMove extends JPanel implements KeyListener { public static int WIDTH=800; public static int HEIGHT=600; public static int SPEED=6; private Font titleFont, regularFont; private Monster fred; private int code=0; public MonsterMove() { //initialize variables here... titleFont = new Font("Roman", Font.BOLD, 18); regularFont = new Font("Helvetica", Font.PLAIN, 12); fred = new Monster("pinky.gif"); } public static void main(String[] args) { MonsterMove app= new MonsterMove(); JFrame window = new JFrame("My Generic Application"); window.setSize(WIDTH, HEIGHT); window.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); window.getContentPane().add(app); window.addKeyListener(app); //window.pack(); window.setVisible(true); } public void paintComponent(Graphics g){ super.paintComponent(g); g.setColor(Color.WHITE); g.fillRect(0, 0, getWidth(),getHeight()); g.setColor(Color.BLUE); g.setFont(titleFont); g.drawString("My Generic Application", 20, 20); g.setColor(Color.BLACK); g.setFont(regularFont); g.drawString("Version 1.0", 20, 40); fred.draw(g); g.drawString("code = "+code, 20, 140); } // update is a workaround to cure Windows screen flicker problem public void update(Graphics g){ paint(g); } // These 3 methods need to be declares to implement the KeyListener Interface @Override public void keyTyped(KeyEvent e) {} @Override public void keyPressed(KeyEvent e) { code=e.getKeyCode(); // left=37 // right = 39 // up=38 // down =40 if (code==37) fred.left(SPEED); if (code==39) fred.right(SPEED); if (code==38) fred.up(SPEED); if (code==40) fred.down(SPEED); repaint(); } @Override public void keyReleased(KeyEvent e) {} }
DodgingMonster.java
import java.awt.*; import java.awt.event.*; import javax.swing.*; import java.util.ArrayList; public class DodgingMonster extends JPanel implements KeyListener,ActionListener { public static int WIDTH=800; public static int HEIGHT=600; public static int SPEED=6; private Font titleFont, regularFont; private Monster fred; private ArrayList<Monster> obsticles; private Timer spawnTimer, moveTimer; private int hits, code; public DodgingMonster() { //initialize variables here... titleFont = new Font("Roman", Font.BOLD, 18); regularFont = new Font("Helvetica", Font.PLAIN, 12); fred = new Monster("pinky.gif"); obsticles = new ArrayList<Monster>(); spawnTimer=new Timer(300,this); moveTimer = new Timer(60, this); spawnTimer.start(); moveTimer.start(); } public static void main(String[] args) { DodgingMonster app= new DodgingMonster(); JFrame window = new JFrame("Avoid Obsticles by pressing arrow keys"); window.setSize(WIDTH, HEIGHT); window.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); window.getContentPane().add(app); window.addKeyListener(app); //window.pack(); window.setVisible(true); } public void paintComponent(Graphics g){ super.paintComponent(g); g.setColor(Color.WHITE); g.fillRect(0, 0, getWidth(),getHeight()); g.setColor(Color.BLUE); g.setFont(titleFont); g.drawString("Dodging Monster", 20, 20); g.setColor(Color.BLACK); g.setFont(regularFont); g.drawString("Version 1.0", 20, 40); fred.draw(g); g.drawString("hits = "+hits, 20, 160); g.drawString("obstile count = "+obsticles.size(), 20, 140); for(Monster o: obsticles){ o.draw(g); } } // update is a workaround to cure Windows screen flicker problem public void update(Graphics g){ paint(g); } // This is the method that responds to the timer's ActionEvent @Override public void actionPerformed(ActionEvent e){ Object source = e.getSource(); if (source==spawnTimer){ obsticles.add(new Monster((int)(WIDTH*Math.random()),20)); }else { for(Monster o: obsticles){ o.down(SPEED); if (o.collides(fred.getRectangle())) hits++; } int i=0; while ( i< obsticles.size()){ if (obsticles.get(i).getY()>HEIGHT) obsticles.remove(i); else i++; } } repaint(); } // These 3 methods need to be declares to implement the KeyListener Interface @Override public void keyTyped(KeyEvent e) {} @Override public void keyPressed(KeyEvent e) { code=e.getKeyCode(); // left=37 // right = 39 // up=38 // down =40 if (code==37) fred.left(SPEED); if (code==39) fred.right(SPEED); if (code==38) fred.up(SPEED); if (code==40) fred.down(SPEED); repaint(); } @Override public void keyReleased(KeyEvent e) {} }