Nifty Scrolling Game
<< Life | LabTrailIndex | Temp Converter >>
This is another nifty assignment from Dave Feinberg at Carnegie Mellon University in 2011.
Click here to see a video of the basic Game
Link to the assignment for instructions, be sure to download the starter code.
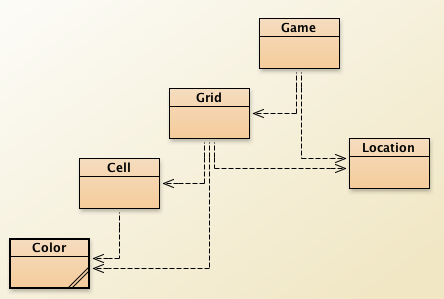
Hints
Step 1
- If you are using Eclipse, and you cannot run the starter code, check the Eclipse preferences(under the Window menu in Windows, the Eclipse menu in OSX). The Java->Build Path should have Project selected (not Folders) for source and output folder:
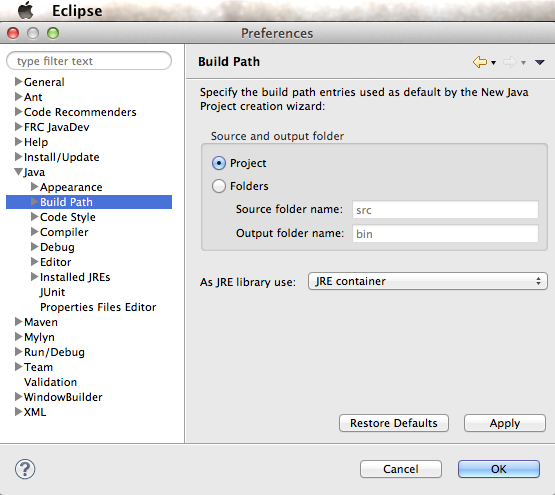
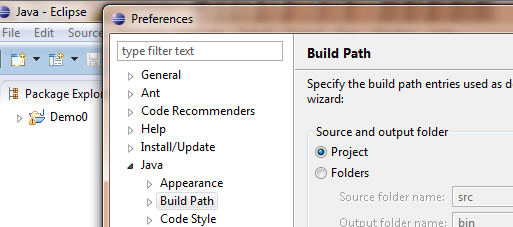
Step 2
- 0 is the top, and bigger numbers are below
- The up arrow key code is 38, down arrow is 40.
- You can find the maximum by using
grid.getNumRows()
- You can store the current location by using
Location loc = new Location( userRow,0 );
first thing. This is important so you can "erase" your old location later. - To "erase" a location, set the image to null:
grid.setImage( loc, null )
- You can get and and store the image to use it later:
String userImageName = grid.getImage( loc )
- You can see if the current
Location
and the nextLocation
are the same:loc.equals( nextLoc )
Step 3
- To choose a random row:
int row = (int)(grid.getNumRows()*Math.random());
- To choose something 20% of the time, you can use an if statement:
if ( Math.random < 0.2 )
- To find the last column:
int lastCol = grid.getNumCols()-1;
- To make an avoidable object:
grid.setImage(loc, "avoid.gif");
- To make a gettable object:
grid.setImage(loc, "get.gif");
- To test your code, make sure your
test
method looks like:public static void test() { Game game = new Game(); game.populateRightEdge(); }
- Be sure to run it a number of times to make sure there are no "index out of bounds" trouble and that you get both A and G objects.
Step 4
- Be sure to not move the user. One way is to treat the first column separately, making note of
userRow
for (int r=0; r<grid.getNumRows();r++) { if (r!=userRow){ Location next = new Location(r,1); grid.setImage(new Location(r,0), grid.getImage(next)); } }
- Use a nested
for
loop to go through each location in all but the first column. - To make sure to avoid destroying things accidentally, Make the outer (first) loop the columns, working toward
grid.getNumCols()-1
for (int c= 1 ; c<grid.getNumCols()-1; c++) for (int r = 0 ; r< grid.getNumRows(); r++){
- Treat the last row separately, making sure it is all
null
(See Hint for Step 2, number 5) - To test buy "running the game", make sure your
test
method looks like:public static void test() { Game game = new Game(); game.play(); }
Step 7
- One idea for the
updateTitle
is to include how many times you've been hitgrid.setTitle("Game: " + getScore()+ " Hit: "+timesAvoid+" times");
Step 8
- One idea is to end when you've been hit 3 times:
return timesAvoid>2 ;
Step 9
Now that the game is functional, you want to be creative. Pick a theme, change the size and colors and graphics. You can see some examples here.
- Look in the
Grid
class to see things at your disposal like anypublic
method. - This project has its own
Color
class, so don't' importjava.awt.Color
! Here is an example:grid.setColor(loc, new Color(255,0,0));
. The 3 parameters are red, green and blue, where 0 is none and 255 is all. You can use the ColorMixer Applet to find the right numbers for the color you want. For example, you can change the background from black to brown with this in theGame
constructor:Color brown = new Color(140,80,0); for(int r=0; r<grid.getNumRows();r++) for (int c=0; c<grid.getNumCols();c++) { Location loc = new Location(r,c); grid.setColor(loc, brown); }
- You can stop the game to send an alert with
grid.showMessageDialog("you were hit!");
- You can change the lame graphics by making your own gif ( or png) files instead of the "user.gif" "avoid.gif" and "get.gif" and in fact SHOULD. You can have more than one type of good thing or bad thing. I like using GIMP, but any graphic editor will do. Its works well if you use a gif or a png file that is square and has a transparent background.