Coin And Purse World
<< Custom ActorWorld that Reports the Furthest Bug | GridworldTrailIndex | ChickenBugs >>
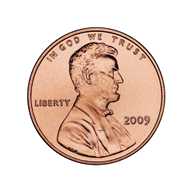
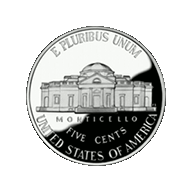
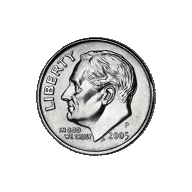
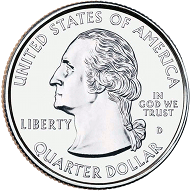
Use these gif files in the same directory as the byte code files of your CoinActor
or CoinCritter
, the difference being if you was to override the act
method, or follow the Critter
procedure of changing one all all five of the Critter's act method.
The Horstmann text has a Purse
class in chapter 7, so I thought this would be a good way to build upon that to mix together the topics of Gridworld, Interfaces and Subclasses. Here we make a subclass of ActorWorld called PurseWorld which will contain different kinds of CoinActor
like Penny
, Nickel
, Dime
and Quarter
. You can also add a Rock
or Bug
to your PurseWorld, and it should still show the correct value of the purse, because it uses a Counter
class that can get information from Countable
Actors, and ignore the others. This way the Purse can have any Actor
in it, but only report the number and the value of the coins, ignoring any other disgusting creature you might have in your purse.
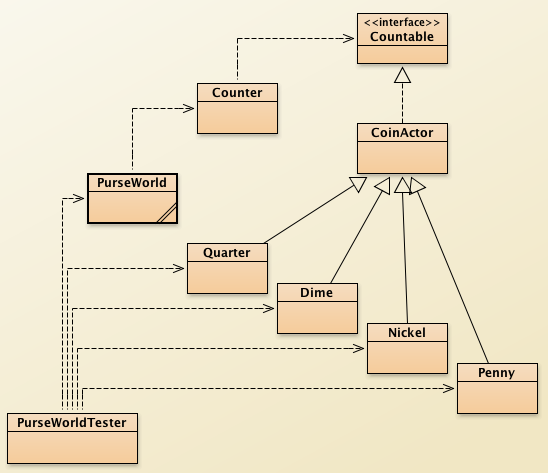
PurseWorldTester.java
import info.gridworld.actor.*; public class PurseWorldTester { public static void main (String[] args) { PurseWorld purse = new PurseWorld(5,5); purse.add(new Quarter(2005) ); purse.add(new Quarter(2005) ); purse.add(new Dime(2005) ); purse.add(new Nickel(2005) ); purse.add(new Penny(2005) ); purse.add(new Penny(2005) ); purse.add(new Rock() ); purse.show(); } }
PurseWorld.java
import java.awt.Color; import java.util.ArrayList; import info.gridworld.actor.*; import info.gridworld.grid.*; public class PurseWorld extends ActorWorld { public PurseWorld(int rows, int cols){ super(); this.setGrid(new BoundedGrid<Actor>(rows,cols)); updateMessage(); } public void step() { updateMessage(); super.step(); } public void updateMessage() { Counter cpa=new Counter(); ArrayList<Actor> actors = getActors(); for(Actor a:actors) cpa.add(a); setMessage(cpa.getCount()+" coins, total is "+cpa.getValue() ); } public ArrayList<Actor> getActors(){ ArrayList<Actor> actors=new ArrayList<Actor>(); Grid<Actor> gr = getGrid(); ArrayList<Location> locs= gr.getOccupiedLocations(); for (Location loc: locs){ Actor a = gr.get(loc); actors.add(a); } return actors; } public void add(Actor a){ super.add(a); updateMessage(); } public void add(Location loc, Actor a){ super.add(loc,a); updateMessage(); } }
Countable.java
interface Countable { double getDollarValue(); }
Counter.java
public class Counter { private int count; private double value; public Counter() { count=0; value=0; } public void add(Object thing){ if (thing instanceof Countable) { count++; value+=((Countable)thing).getDollarValue(); } } public double getValue(){ return value;} public double getCount(){ return count;} public double getAverage() {return value/count;} }
CoinActor.java
import info.gridworld.actor.*; import info.gridworld.grid.*; import java.awt.Color; public class CoinActor extends Actor implements Countable { // instance variables - replace the example below with your own private int cents, year; //worth of coin in pennies, year of minting /** * Constructor for objects of class CoinActor */ public CoinActor(int cents, int year) { super(); this.cents=cents; this.year=year; setColor(null); } public CoinActor(int cents) { this(cents, 2011); } public CoinActor(){ this(1,2011); } public int getCents(){ return cents;} public int getYear(){return year;} public double getDollarValue() { return getCents()/100.0; } public String toString(){ return year+" "+getClass().getName()+ " worth "+cents+" cents"; } public void act() { //First we tarnish Color c = getColor(); if (c==null) c = new Color(255,255,255); setColor(c.darker() ); //Now we fall if we can Grid<Actor> gr = getGrid(); if(gr == null ) return; Location loc =getLocation(); Location next=loc.getAdjacentLocation(Location.SOUTH); if(!gr.isValid(next)) return; Actor occupant=gr.get(next); if (occupant==null ) moveTo(next); } }
Penny.java
public class Penny extends CoinActor { // instance variables - replace the example below with your own /** * Constructor for objects of class Quarter */ public Penny(int year) { super(1, year); } public Penny() { super(1); } }