Chicken Bugs
<< Coin and PurseWorld | GridworldTrailIndex | Mushroom >>
Make a Bug that has a chance of dropping an Egg instead of a Flower. Once that is working, modify the Egg so it has a chance of hatching into a ChickenBug. For extra credit, answer this question: Which came first, the ChickenBug or the Egg? Seriously, all you have to do is to figure out how to make the ChickenBug lay an egg (much like a Bug dropping a Flower), or how an Egg replaces itself with a ChickenBug. Note that this is a Bug and not a Critter, so there is more freedom here. The ChickenBugWorld is a subclass of ActorWorld (not on the AP Exam), and uses some Grid methods to look for the different types of Breeds, and counts how many of each type. You may get ideas in this part of the code for when you are working with Critters, when you need to process actors in the grid. For fun, all breeds have the same probability for hatching, and all the breeds have the same gestation period... you will have different winners each time you run it.
Things to Try
- complete the Egg and ChickenBug classes so it works like the movie
- change the size of the world
- change the gestation period
- change the probability for hatching
Click here for a Quicktime Movie
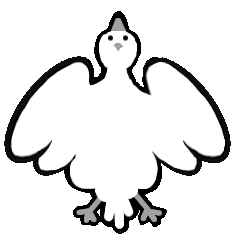
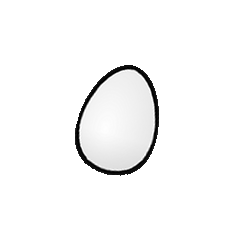
Egg.java
import java.awt.Color; import info.gridworld.actor.Rock; import info.gridworld.grid.Grid; import info.gridworld.grid.Location; public class Egg extends Rock { private static final int GESTATION=7; private static final double probability = 0.50; private int age; private String breed; public Egg(){ setColor(Color.WHITE); age=0; breed="Leghorn"; } public Egg(Color c, String b){ setColor(c); age=0; breed=b; } public void act(){ age++; if (age==GESTATION && Math.random()<probability){ //hatch - your code here - see how Bugs drop flowers for a hint } if (age>GESTATION) this.removeSelfFromGrid(); super.act(); } public String getBreed() { return breed; } public void setBreed(String breed) { this.breed = breed; } }
ChickenBug.java
import java.awt.Color; import info.gridworld.actor.Actor; import info.gridworld.actor.Bug; import info.gridworld.grid.Grid; import info.gridworld.grid.Location; public class ChickenBug extends Bug { private static final double FERTILITY=0.5; private String breed; public ChickenBug() { setBreed("Leghorn"); setColor(Color.WHITE); } public ChickenBug(Color c, String b) { setColor(c); setBreed(b); } /** * overload the move method with a new * one that drops Egg based on * PROBABILITY */ public void move() { Grid<Actor> gr = getGrid(); if (gr == null) return; Location loc = getLocation(); Location next = loc.getAdjacentLocation(getDirection()); if (gr.isValid(next)) moveTo(next); else removeSelfFromGrid(); if (Math.random()<FERTILITY){ //lay an egg //your code here - See how Bugs drop flowers for a hint } } public String getBreed() { return breed; } public void setBreed(String breed) { this.breed = breed; } }
ChickenBugWorld.java
import java.awt.Color; import java.util.ArrayList; import info.gridworld.actor.*; import info.gridworld.grid.BoundedGrid; import info.gridworld.grid.Grid; import info.gridworld.grid.Location; public class ChickenBugWorld extends ActorWorld { public static void main (String[] args) { ChickenBugWorld myWorld = new ChickenBugWorld(5,5); myWorld.setMessage("Chicken Bug World--Press Step or Run to begin"); myWorld.add(new Location(3,4), new Egg()); myWorld.add(new Location(0,2), new Rock()); myWorld.add(new Location(1,1), new ChickenBug()); ChickenBug greeny=new ChickenBug(Color.GREEN, "Marsh Daisy"); myWorld.add(new Location (1,0), greeny); ChickenBug molly=new ChickenBug(new Color(200,75,20), "Rhodebar"); myWorld.add(new Location(3,3), molly); myWorld.show(); } public ChickenBugWorld(int rows, int cols){ super(); this.setGrid(new BoundedGrid<Actor>(rows,cols)); updateMessage(); } public void step() { updateMessage(); super.step(); } public void updateMessage() { ArrayList<String> types=new ArrayList<String>(); ArrayList<ChickenBug> birds = getChickenBugs(); for(ChickenBug c:birds){ if (!types.contains(c.getBreed()) ){ types.add(c.getBreed()); } } int[] count=new int[types.size()]; for(ChickenBug c:birds) for (int i=0; i<types.size(); i++) if (c.getBreed().equals(types.get(i))) count[i]++; String message=""; for (int i=0;i<types.size();i++) message+=" "+count[i]+" "+types.get(i)+";"; setMessage(message); } public ArrayList<ChickenBug> getChickenBugs(){ Grid<Actor> gr = getGrid(); ArrayList<ChickenBug> foul = new ArrayList<ChickenBug>(); ArrayList<Location> locs= gr.getOccupiedLocations(); for (Location loc: locs){ Actor a = gr.get(loc); if (a instanceof ChickenBug) foul.add((ChickenBug)a); } return foul; } }