Missile
<< RockHound | GridworldTrailIndex | PigeonCritter >>
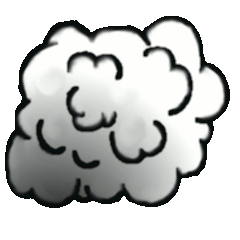
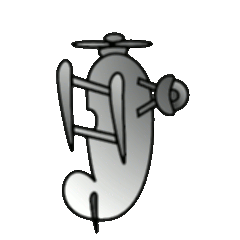
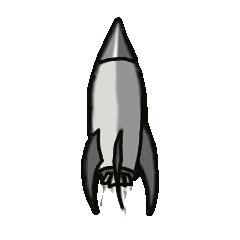
See a Video of MissileCritter in Action
First we need to build an Explosion
class that will initially be red, then become Light_Gray, then become white, and then disappear.
Next make a PlaneCritter
class that will either travel from left to right or right to left along a row. It will need a boolean
attribute called hit
, so that if a missile is in a neighboring position, it will know to explode itself and tell the missile to remove itself from the grid. The PlaneCritter
should behave so that when it is hit, it will replace itself with an Explosion
. If a PlaneCritter
flies into an Explosion
, it should make the Explosion
disappear.
if the forward location is invalid or is occupied by another PlaneCritter
, it should change its direction 180 degrees (Location.HALF_CIRCLE
).
Finally make a MissileCritter
that will target a PlaneCritter
, by turning itself toward the PlaneCritter
and moving to the adjacent location one row up from its current row that is in the direction of the target. The MissileCritter
should remove itself from the grid if it is currently on the top row.
Hints:
- Remember to heed the required post-conditions! The state of all actors should be unchanged in the
getActors()
,getMoveLocations()
, andselectMoveLocation
methods. - The
processActors()
method cannot change the state of any actors except for itself and and any actors in theactors
ArrayList parameter (hint: use thegetActors()
method to add any actor to the list of actors!) - The
makeMove
method can only change the state of the actors at the old and new locations. getMoveLocations()
should return something, even if it is an emptyArrayList<Location>
selectMoveLocation
can see if there are any Locations to move to... if it is empty list, it can return anull
location, so thatmakeMove
can recieve thenull
location, and remove itself from the grid.- Having trouble with replacing the plane with an explosion? Record the grid and location of the plane before you remove it from the grid! Still having troubles? Don't add the explosion before you first remove the plane!
MissileCritterRunner.java
import info.gridworld.actor.*; import info.gridworld.grid.*; public class MissleCritterRunner { public static void main(String[] args) { ActorWorld world=new ActorWorld(); world.setGrid(new BoundedGrid<Actor>(8,10)); world.add(new Location(5,4), new Explosion()); PlaneCritter p1=new PlaneCritter(); p1.setDirection(Location.WEST); PlaneCritter p2=new PlaneCritter(); world.add(new Location (2,0),p2); world.add(new Location(1,9), p1); world.add(new Location(7,9),new MissileCritter()); world.setMessage("You can add more planes and missiles buy clicking on a grid location."); world.show(); } }