Picture Lab Activity 4
<< Picture Lab Activity 3 | Picture Lab | Picture Lab Activity 5 >>
Two-dimensional arrays in Java
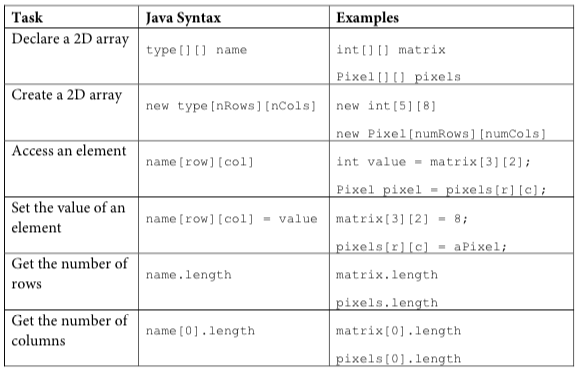
To loop through the values in a 2D array you must have two indexes. One index is used to change the row index and one is used to change the column index. You can use nested loops, which is one for
loop inside of another, to loop through all the values in a 2D array.
Here is a method in the IntArrayWorker
class that totals all the values in a 2D array of integers in a private instance variable (field in the class) named matrix
. Notice the nested for
loop and how it uses matrix.length
to get the number of rows and matrix[0].length
to get the number of columns. Since matrix[0]
returns the inner array in a 2D array, you can use matrix[0].length
to get the number of columns.
public int getTotal() { int total = 0; for (int row = 0; row < matrix.length; row++) { for (int col = 0; col < matrix[0].length; col++) { total = total + matrix[row][col]; } } return total; }
Because Java two-dimensional arrays are actually arrays of arrays, you can also get the total using nested "for-each" loops as shown in getTotalNested
below. The outer loop will loop through the outer array (each of the rows) and the inner loop will loop through the inner array (columns in that row).
You can use a nested for-each loop whenever you want to loop through all items in a 2D array and you don't need to know the row index or column index.
public int getTotalNested() { int total = 0; for (int[] rowArray : matrix) { for (int item : rowArray) { total = total + item; } } return total; }
Exercises
- Write a
getCount(int i)
method in theIntArrayWorker
class that returns the count of the number of times the integer valuei
is found in the matrix. There is already a method to test this inIntArrayWorkerTester
. Just uncomment the methodtestGetCount()
(around line 25) and the call to it in the main method ofIntArrayWorkerTester
. - Write a
getLargest
method in theIntArrayWorker
class that returns the largest value in the matrix. There is already a method to test this inIntArrayWorkerTester
. Just uncomment the methodtestGetLargest()
(around line 55) and the call to it in the main method ofIntArrayWorkerTester
. - Write a
getColTotal(int col)
method in theIntArrayWorker
class that returns the total of all integers in a specified column. There is already a method to test this inIntArrayWorkerTester
(around line 80). Just uncomment the methodtestGetColTotal()
and the call to it in the main method ofIntArrayWorkerTester
.